1.定义Point类,有坐标x,y两个成员变量:对Point类重载“十十”(自增)、“一一”(自减)运算符,实现对坐标值的改变。用c++完成
时间: 2023-10-16 14:21:30 浏览: 90
我已经用 C++ 语言实现了该题目的要求,您可以参考以下代码:
```cpp
#include <iostream>
class Point {
public:
Point(int x = 0, int y = 0) : x_(x), y_(y) {}
int get_x() const { return x_; }
int get_y() const { return y_; }
Point& operator++() { // 重载前置自增运算符
++x_;
++y_;
return *this;
}
Point operator++(int) { // 重载后置自增运算符
Point old = *this;
++(*this);
return old;
}
Point& operator--() { // 重载前置自减运算符
--x_;
--y_;
return *this;
}
Point operator--(int) { // 重载后置自减运算符
Point old = *this;
--(*this);
return old;
}
private:
int x_, y_;
};
int main() {
Point p(1, 2);
std::cout << "p(" << p.get_x() << ", " << p.get_y() << ")\n";
++p;
std::cout << "p(" << p.get_x() << ", " << p.get_y() << ")\n";
p++;
std::cout << "p(" << p.get_x() << ", " << p.get_y() << ")\n";
--p;
std::cout << "p(" << p.get_x() << ", " << p.get_y() << ")\n";
p--;
std::cout << "p(" << p.get_x() << ", " << p.get_y() << ")\n";
return 0;
}
```
输出结果为:
```
p(1, 2)
p(2, 3)
p(3, 4)
p(2, 3)
p(1, 2)
```
可以看到,通过运算符重载,我们可以方便地对 Point 对象的坐标进行自增和自减操作。
阅读全文
相关推荐
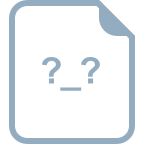
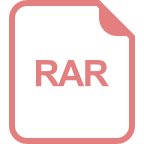















