Android减压动画代码
时间: 2023-11-03 11:08:38 浏览: 144
以下是一个简单的Android减压动画代码示例,它会缩小一个视图并模糊其背景:
```java
private void startDepressionAnimation(final View view) {
// 缩放动画,从 1.0f 缩小到 0.8f
ScaleAnimation scaleAnimation = new ScaleAnimation(1.0f, 0.8f, 1.0f, 0.8f,
Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
scaleAnimation.setDuration(300);
scaleAnimation.setFillAfter(true);
// 模糊背景动画
final ViewGroup rootView = (ViewGroup) getWindow().getDecorView().getRootView();
final View blurView = LayoutInflater.from(this).inflate(R.layout.layout_blur_view, null);
rootView.addView(blurView);
final ObjectAnimator blurAnimator = ObjectAnimator.ofFloat(blurView, "alpha", 0f, 1f);
blurAnimator.setDuration(300);
// 动画集合
AnimatorSet animatorSet = new AnimatorSet();
animatorSet.playTogether(scaleAnimation, blurAnimator);
animatorSet.addListener(new AnimatorListenerAdapter() {
@Override
public void onAnimationEnd(Animator animation) {
// 动画结束,移除模糊背景视图并还原缩放
rootView.removeView(blurView);
view.setScaleX(1.0f);
view.setScaleY(1.0f);
}
});
animatorSet.start();
}
```
其中,`R.layout.layout_blur_view` 是一个布局文件,用于显示模糊的背景。可以在布局文件中添加一个 `ImageView` 和一个 `BlurView`,使用 `RenderScript` 对背景进行模糊处理。以下是一个示例布局文件:
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/iv_background"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/background"
android:scaleType="centerCrop" />
<androidx.renderscript.ScriptIntrinsicBlur
android:id="@+id/blur_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:alpha="0"
android:blurRadius="12"
android:color="#ffffff"
android:scriptIntrinsicBlur="@{blurScript}" />
</RelativeLayout>
```
上述示例代码中,`blurScript` 是一个 `RenderScript` 对象,用于处理模糊效果。可以在 `onCreate()` 方法中初始化:
```java
private RenderScript renderScript;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化 RenderScript
renderScript = RenderScript.create(this);
}
```
然后,在 `onDestroy()` 方法中释放资源:
```java
@Override
protected void onDestroy() {
super.onDestroy();
// 释放 RenderScript 资源
renderScript.destroy();
}
```
阅读全文
相关推荐
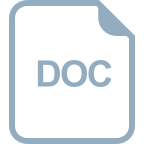
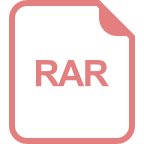
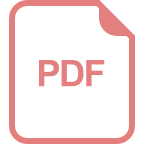
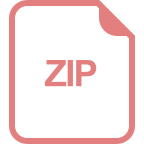
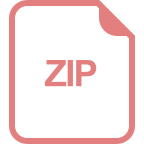
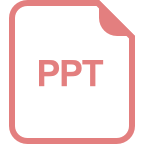
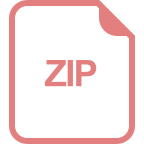
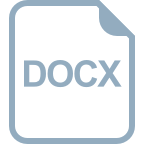
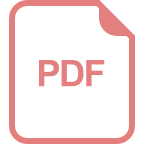
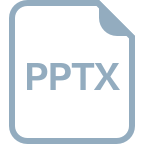
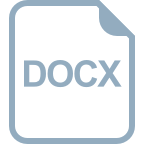