print("%4d%s"%(n, line), end='') 什么意思
时间: 2023-09-24 16:11:15 浏览: 83
这是一个 Python 的字符串格式化输出语句,其中:
- `%4d` 表示将一个整数(d)格式化成长度为 4 的字符串,如果整数不足 4 位,则在左侧补空格;
- `%s` 表示将一个字符串(s)格式化成字符串本身;
- `%(n, line)` 表示将格式化字符串中的第一个占位符 `%4d` 替换成变量 n 的值,第二个占位符 `%s` 替换成变量 line 的值。
最后,`end=''` 表示输出格式不要换行。
相关问题
# 导入所需的库 import cv2 import time import numpy as np # 加载OpenPose模型 net = cv2.dnn.readNetFromTensorflow("C:\Users\1\Desktop\onem.jpg") # 配置OpenCV窗口 cv2.namedWindow("OpenPose Demo", cv2.WINDOW_NORMAL) # 加载要测试的图像 image = cv2.imread("C:\Users\1\Desktop\onem.jpg") # 获取图像的宽和高 width = image.shape[1] height = image.shape[0] # 创建一个4D blob,将图像传递给OpenPose模型 blob = cv2.dnn.blobFromImage(image, 1.0 / 255, (368, 368), (0, 0, 0), swapRB=False, crop=False) # 设置输入blob net.setInput(blob) # 运行前向传递,获取OpenPose模型的输出 start = time.time() output = net.forward() end = time.time() # 输出运行时间 print("OpenPose took {:.2f} seconds".format(end - start)) # 获取输出blob的大小 H = output.shape[2] W = output.shape[3] # 创建一个空列表,用于存储检测到的人体姿态 points = [] # 遍历检测到的人体关键点 for i in range(18): # 提取x和y坐标 probMap = output[0, i, :, :] minVal, prob, minLoc, point = cv2.minMaxLoc(probMap) # 如果概率大于阈值,则将其添加到列表中 if prob > 0.1: x = int((width * point[0]) / W) y = int((height * point[1]) / H) points.append((x, y)) # 绘制检测到的人体姿态 for i, point in enumerate(points): cv2.circle(image, point, 5, (0, 255, 255), thickness=-1, lineType=cv2.FILLED) cv2.putText(image, "{}".format(i), point, cv2.FONT_HERSHEY_SIMPLEX, 1, (0, 0, 255), 2, lineType=cv2.LINE_AA) # 显示结果 cv2.imshow("OpenPose Demo", image) cv2.waitKey(0) cv2.destroyAllWindows()
这段代码是一个使用OpenPose模型检测人体姿态的示例。它的主要步骤包括:
1. 导入所需的库,包括OpenCV、NumPy和时间库。
2. 加载OpenPose模型。
3. 加载要测试的图像。
4. 创建一个4D blob,将图像传递给OpenPose模型。
5. 运行前向传递,获取OpenPose模型的输出。
6. 遍历检测到的人体关键点,并将概率大于阈值的点添加到列表中。
7. 绘制检测到的人体姿态,并在每个关键点上添加标记。
8. 显示结果图像。
你可以根据自己的需要对这段代码进行修改和调整,例如更改输入图像的路径、更改姿态检测的阈值等。同时,也可以了解更多关于OpenPose模型的知识,以更好地理解这段代码的实现。
设计Rinex O文件和Rinex N文件读取类,实现对Rinex文件内容的读取和显示.c+=
以下是一个简单的 Rinex O 文件读取类和 Rinex N 文件读取类的实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
class RinexOReader {
public:
RinexOReader(string filename) {
ifstream input(filename);
if (!input.is_open()) {
cerr << "Error: Could not open file " << filename << endl;
exit(1);
}
string line;
while (getline(input, line)) {
if (line.empty()) continue; // skip empty lines
if (line.find("END OF HEADER") != string::npos) break; // end of header
header_lines.push_back(line);
}
while (getline(input, line)) {
if (line.empty()) continue; // skip empty lines
double time;
int prn;
double phase, doppler, snr;
sscanf(line.c_str(), "%lf %d %lf %lf %lf", &time, &prn, &phase, &doppler, &snr);
observations.push_back({time, prn, phase, doppler, snr});
}
input.close();
}
void print_header() {
for (auto line : header_lines) {
cout << line << endl;
}
}
void print_observations() {
for (auto obs : observations) {
printf("%.3lf %2d %10.3lf %10.3lf %5.1lf\n", obs.time, obs.prn, obs.phase, obs.doppler, obs.snr);
}
}
private:
struct Observation {
double time;
int prn;
double phase;
double doppler;
double snr;
};
vector<string> header_lines;
vector<Observation> observations;
};
class RinexNReader {
public:
RinexNReader(string filename) {
ifstream input(filename);
if (!input.is_open()) {
cerr << "Error: Could not open file " << filename << endl;
exit(1);
}
string line;
while (getline(input, line)) {
if (line.empty()) continue; // skip empty lines
if (line.find("END OF HEADER") != string::npos) break; // end of header
header_lines.push_back(line);
}
while (getline(input, line)) {
if (line.empty()) continue; // skip empty lines
int year, month, day, hour, minute;
double second;
char type;
int nsats;
sscanf(line.c_str(), "%4d %2d %2d %2d %2d %lf %c %d", &year, &month, &day, &hour, &minute, &second, &type, &nsats);
epochs.push_back({year, month, day, hour, minute, second, type, nsats});
for (int i = 0; i < nsats; i++) {
getline(input, line);
if (line.empty()) continue; // skip empty lines
int prn;
double pseudorange, carrierphase, doppler, snr;
sscanf(line.c_str(), "%2d %14lf %14lf %9lf %5lf", &prn, &pseudorange, &carrierphase, &doppler, &snr);
observations.push_back({prn, pseudorange, carrierphase, doppler, snr});
}
}
input.close();
}
void print_header() {
for (auto line : header_lines) {
cout << line << endl;
}
}
void print_epochs() {
for (auto epoch : epochs) {
printf("%4d-%02d-%02d %02d:%02d:%06.3lf %c %2d\n", epoch.year, epoch.month, epoch.day, epoch.hour, epoch.minute, epoch.second, epoch.type, epoch.nsats);
for (int i = 0; i < epoch.nsats; i++) {
auto obs = observations.front();
observations.erase(observations.begin());
printf(" %2d %14.3lf %14.3lf %9.3lf %5.1lf\n", obs.prn, obs.pseudorange, obs.carrierphase, obs.doppler, obs.snr);
}
}
}
private:
struct Epoch {
int year;
int month;
int day;
int hour;
int minute;
double second;
char type;
int nsats;
};
struct Observation {
int prn;
double pseudorange;
double carrierphase;
double doppler;
double snr;
};
vector<string> header_lines;
vector<Epoch> epochs;
vector<Observation> observations;
};
int main() {
RinexOReader o_reader("example.17o");
o_reader.print_header();
o_reader.print_observations();
RinexNReader n_reader("example.17n");
n_reader.print_header();
n_reader.print_epochs();
return 0;
}
```
其中,RinexOReader 类用于读取 Rinex O 文件,RinexNReader 类用于读取 Rinex N 文件。这两个类都包含一个成员函数 print_header() 和一个成员函数 print_observations(),用于分别显示文件头和观测值内容。
在 RinexOReader 类中,我们使用了一个结构体 Observation,表示一个观测值,包括时间、卫星编号、相位观测值、多普勒观测值和信噪比。我们使用了 vector<Observation> 类型的成员变量 observations 存储所有的观测值。
在 RinexNReader 类中,我们使用了两个结构体 Epoch 和 Observation,分别表示一个历元和一个观测值,其中历元包括时间、历元类型(如 P 表示伪距历元,L 表示相位历元)、卫星数目等信息,使用 vector<Epoch> 类型的成员变量 epochs 存储所有的历元,每个历元对应一个或多个观测值,使用 vector<Observation> 类型的成员变量 observations 存储所有的观测值。
此外,我们在读取每一行数据时,使用了 sscanf 函数将字符串转换为对应的数据类型。注意,sscanf 函数的第一个参数为字符串,第二个参数为格式化字符串,后面的参数为需要赋值的变量的地址。
最后,在 main 函数中,我们分别创建了 RinexOReader 和 RinexNReader 的实例,并调用它们的成员函数来显示文件内容。
阅读全文
相关推荐
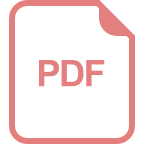
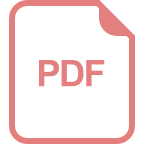
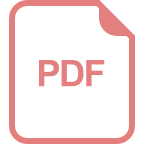
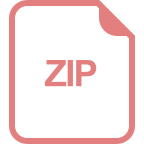
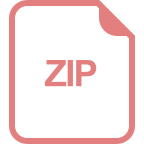
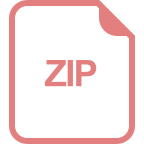
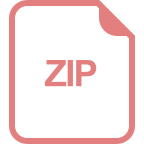
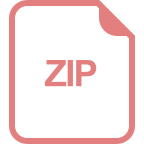
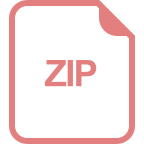
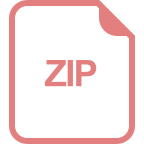
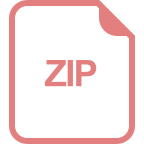
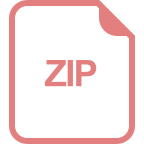
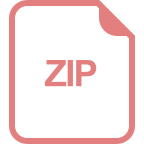