改正下面代码错误:using Org.BouncyCastle.Math; using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace WindowsFormsApp1 { public partial class Form7 : Form { public Form7() { InitializeComponent(); } private void btnEncrypt_Click(object sender, EventArgs e) { // 生成公钥和私钥 BigInteger p = BigInteger.Parse("857504083339712752489993810777"); BigInteger q = BigInteger.Parse("1029224947942998075080348647219"); BigInteger n = p * q; BigInteger phi = (p - 1) * (q - 1); BigInteger e = 65537; BigInteger d = ModInverse(e, phi); // 加密数据 BigInteger message = BigInteger.Parse(txtData.Text); BigInteger encrypted = ModPow(message, e, n); // 解密数据 BigInteger decrypted = ModPow(encrypted, d, n); // 显示结果 txtEncrypted.Text = encrypted.ToString(); txtDecrypted.Text = decrypted.ToString(); } // 求模反元素 public static BigInteger ModInverse(BigInteger a, BigInteger m) { BigInteger m0 = m; BigInteger y = 0, x = 1; if (m == 1) return 0; while (a > 1) { BigInteger q = a / m; BigInteger t = m; m = a % m; a = t; t = y; y = x - q * y; x = t; } if (x < 0) x += m0; return x; } // 模幂运算 public static BigInteger ModPow(BigInteger b, BigInteger e, BigInteger m) { BigInteger result = 1; while (e > 0) { if ((e & 1) == 1) result = (result * b) % m; e >>= 1; b = (b * b) % m; } return result; } } }
时间: 2024-02-10 17:16:08 浏览: 24
在代码中,变量名与方法名重复了,需要修改。具体来说,把变量名 `e` 改成 `eValue`,避免与 `ModPow` 方法中的 `e` 混淆;把变量名 `encrypted` 改成 `encryptedMessage`,使其更加具有描述性。修改后的代码如下:
```csharp
using Org.BouncyCastle.Math;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form7 : Form
{
public Form7()
{
InitializeComponent();
}
private void btnEncrypt_Click(object sender, EventArgs e)
{
// 生成公钥和私钥
BigInteger p = BigInteger.Parse("857504083339712752489993810777");
BigInteger q = BigInteger.Parse("1029224947942998075080348647219");
BigInteger n = p * q;
BigInteger phi = (p - 1) * (q - 1);
BigInteger eValue = 65537;
BigInteger d = ModInverse(eValue, phi);
// 加密数据
BigInteger message = BigInteger.Parse(txtData.Text);
BigInteger encryptedMessage = ModPow(message, eValue, n);
// 解密数据
BigInteger decryptedMessage = ModPow(encryptedMessage, d, n);
// 显示结果
txtEncrypted.Text = encryptedMessage.ToString();
txtDecrypted.Text = decryptedMessage.ToString();
}
// 求模反元素
public static BigInteger ModInverse(BigInteger a, BigInteger m)
{
BigInteger m0 = m;
BigInteger y = 0, x = 1;
if (m == 1)
return 0;
while (a > 1)
{
BigInteger q = a / m;
BigInteger t = m;
m = a % m;
a = t;
t = y;
y = x - q * y;
x = t;
}
if (x < 0)
x += m0;
return x;
}
// 模幂运算
public static BigInteger ModPow(BigInteger b, BigInteger e, BigInteger m)
{
BigInteger result = 1;
while (e > 0)
{
if ((e & 1) == 1)
result = (result * b) % m;
e >>= 1;
b = (b * b) % m;
}
return result;
}
}
}
```
相关推荐
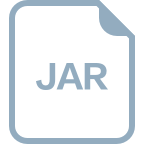















