python统计字典有多少k
时间: 2024-02-03 11:45:43 浏览: 167
可以使用Python内置函数`len()`来统计字典中键的数量,例如:
```python
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
num_keys = len(my_dict)
print("字典中有", num_keys, "个键")
```
运行结果为:
```
字典中有 3 个键
```
因此,上述字典中有3个k。
相关问题
python统计字典中的最大最小值
可以使用Python内置的max()和min()函数。示例代码如下:
```python
my_dict = {'apple': 5, 'banana': 2, 'grape': 10, 'orange': 3}
max_value = max(my_dict.values())
max_key = [k for k, v in my_dict.items() if v == max_value][0]
min_value = min(my_dict.values())
min_key = [k for k, v in my_dict.items() if v == min_value][0]
print(f"The maximum value in the dictionary is {max_value}, associated with the key '{max_key}'")
print(f"The minimum value in the dictionary is {min_value}, associated with the key '{min_key}'")
```
输出:
```
The maximum value in the dictionary is 10, associated with the key 'grape'
The minimum value in the dictionary is 2, associated with the key 'banana'
```
python统计字典的value出现次数,并返回新的字典key为原value,value为出现次数的新字典
你可以使用 Python 的 `collections` 模块中的 `Counter` 类来实现该功能。具体代码如下:
```python
from collections import Counter
# 原始字典
original_dict = {'a': 1, 'b': 2, 'c': 2, 'd': 3}
# 统计 value 出现次数
value_counts = Counter(original_dict.values())
# 新的字典
new_dict = {k: value_counts[v] for k, v in original_dict.items()}
print(new_dict) # 输出: {'a': 1, 'b': 2, 'c': 2, 'd': 1}
```
在上面的代码中,我们首先定义了一个原始的字典 `original_dict`,然后使用 `Counter` 类统计了原始字典中 value 出现的次数,得到了一个计数器对象 `value_counts`。最后,我们通过遍历原始字典中的每一个键值对,将原始字典中的 value 替换为其出现次数,生成了一个新的字典 `new_dict`。
阅读全文
相关推荐






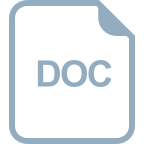









