c语言中实现:统计一个文本文件中,每个字母出现的次数(不区分大小写),要求以哈希表的方式实现。
时间: 2023-06-24 15:06:03 浏览: 141
哈希表是一种用于快速查找的数据结构,可以用来统计每个字母出现的次数。下面是一个简单的 C 代码示例,使用哈希表实现对字母出现次数的统计:
```c
#include <stdio.h>
#include <ctype.h>
#define TABLE_SIZE 26
typedef struct Node {
char key;
int value;
struct Node* next;
} Node;
typedef struct {
Node* table[TABLE_SIZE];
} HashTable;
int hash(char key) {
return tolower(key) - 'a';
}
void insert(HashTable* hashtable, char key) {
int index = hash(key);
Node* node = hashtable->table[index];
while (node != NULL) {
if (node->key == key) {
node->value++;
return;
}
node = node->next;
}
node = (Node*) malloc(sizeof(Node));
node->key = key;
node->value = 1;
node->next = hashtable->table[index];
hashtable->table[index] = node;
}
void print(HashTable* hashtable) {
for (int i = 0; i < TABLE_SIZE; i++) {
Node* node = hashtable->table[i];
while (node != NULL) {
printf("%c: %d\n", node->key, node->value);
node = node->next;
}
}
}
int main() {
HashTable hashtable = {0};
FILE* fp = fopen("text.txt", "r");
char c;
while ((c = fgetc(fp)) != EOF) {
if (isalpha(c)) {
insert(&hashtable, c);
}
}
fclose(fp);
print(&hashtable);
return 0;
}
```
在这个例子中,我们定义了一个 `Node` 结构体来存储每个字母及其出现次数,然后使用哈希函数将字母映射到哈希表中的索引位置。如果哈希表中已经存在该字母,则增加其出现次数;否则,创建一个新的节点并插入到哈希表中。
在 `main()` 函数中,我们打开了一个文本文件,并逐字读取其中的内容。当读取到字母时,调用 `insert()` 函数将其插入到哈希表中。最后,调用 `print()` 函数将哈希表中所有节点的内容打印出来。
阅读全文
相关推荐
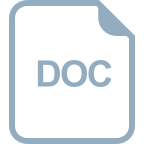
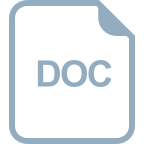
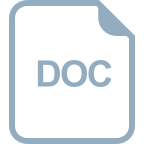


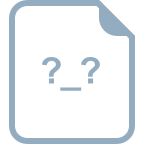
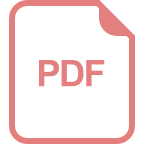
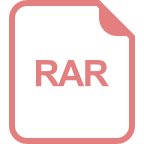
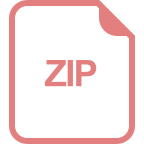
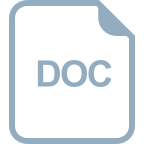
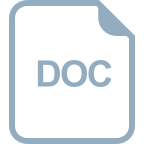
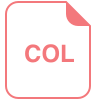
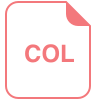
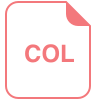




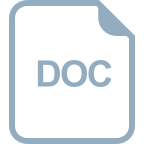