统计一个文本文件中,每个字母出现的次数(不区分大小写),要求以哈希表的方式用C语言实现。
时间: 2023-06-20 11:09:28 浏览: 41
以下是一个简单的C语言程序,用于统计一个文本文件中每个字母出现的次数。它使用了哈希表来存储每个字母的计数,并且不区分大小写。
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define TABLE_SIZE 26
typedef struct node {
char key;
int value;
struct node *next;
} Node;
typedef struct hash_table {
Node *table[TABLE_SIZE];
} HashTable;
int hash(char c) {
return tolower(c) - 'a';
}
void init_table(HashTable *ht) {
for (int i = 0; i < TABLE_SIZE; i++) {
ht->table[i] = NULL;
}
}
void insert(HashTable *ht, char key) {
int index = hash(key);
Node *node = ht->table[index];
while (node != NULL) {
if (node->key == key) {
node->value++;
return;
}
node = node->next;
}
Node *new_node = malloc(sizeof(Node));
new_node->key = key;
new_node->value = 1;
new_node->next = ht->table[index];
ht->table[index] = new_node;
}
void print_table(HashTable *ht) {
for (int i = 0; i < TABLE_SIZE; i++) {
Node *node = ht->table[i];
while (node != NULL) {
printf("%c: %d\n", node->key, node->value);
node = node->next;
}
}
}
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s filename\n", argv[0]);
return 1;
}
FILE *fp = fopen(argv[1], "r");
if (fp == NULL) {
printf("Error opening file %s\n", argv[1]);
return 1;
}
HashTable ht;
init_table(&ht);
char c;
while ((c = fgetc(fp)) != EOF) {
if (isalpha(c)) {
insert(&ht, c);
}
}
fclose(fp);
print_table(&ht);
return 0;
}
```
这个程序首先定义了一个哈希表结构体和一个节点结构体。哈希表使用一个指针数组来存储链表的头指针,每个链表中的节点包含一个键(即字母)和一个值(即出现的次数)。
`hash` 函数用于计算键的哈希值,它将字母转换为小写并将其映射到从 0 到 25 的整数。
`init_table` 函数用于初始化哈希表,它将每个链表的头指针都设置为 NULL。
`insert` 函数用于将一个键插入到哈希表中。如果键已经存在,则将其值加 1;否则,创建一个新节点并将其插入到链表的开头。
`print_table` 函数用于打印哈希表中的所有键和值。
`main` 函数首先检查命令行参数是否正确,并打开指定的文件。然后,它初始化哈希表并遍历文件中的每个字符。如果字符是字母,则将其插入到哈希表中。最后,它关闭文件并打印哈希表中的所有键和值。
相关推荐
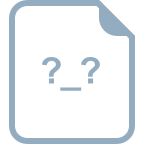














