Spring Boot +mybatis +oracle开发restful风格的查询接口
时间: 2024-01-02 07:03:43 浏览: 180
好的,下面是一个简单的示例:
1. 在 pom.xml 中添加 Spring Boot、MyBatis 和 Oracle JDBC 驱动的依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.3</version>
</dependency>
<dependency>
<groupId>com.oracle.database.jdbc</groupId>
<artifactId>ojdbc8</artifactId>
<version>19.3.0.0</version>
</dependency>
</dependencies>
```
2. 配置 Oracle 数据库连接信息
在 `application.properties` 文件中添加以下配置:
```
spring.datasource.url=jdbc:oracle:thin:@localhost:1521:ORCL
spring.datasource.username=your_username
spring.datasource.password=your_password
spring.datasource.driver-class-name=oracle.jdbc.driver.OracleDriver
```
3. 创建 MyBatis Mapper
在 `src/main/resources` 目录下创建 `mapper` 文件夹,然后创建一个 `UserMapper.xml` 文件。示例代码如下:
```xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.UserMapper">
<select id="getUserById" parameterType="int" resultType="com.example.demo.entity.User">
SELECT * FROM users WHERE id = #{id}
</select>
<select id="getAllUsers" resultType="com.example.demo.entity.User">
SELECT * FROM users
</select>
</mapper>
```
注意:`com.example.demo.entity.User` 是一个简单的实体类,包含 `id`、`name` 和 `email` 三个属性。
4. 创建 MyBatis DAO
在 `src/main/java` 目录下创建 `dao` 文件夹,然后创建一个 `UserDao.java` 接口。示例代码如下:
```java
package com.example.demo.dao;
import com.example.demo.entity.User;
import org.apache.ibatis.annotations.Mapper;
import org.springframework.stereotype.Repository;
import java.util.List;
@Mapper
@Repository
public interface UserDao {
User getUserById(int id);
List<User> getAllUsers();
}
```
5. 创建 Service 和 Controller
在 `src/main/java` 目录下创建 `service` 文件夹,然后创建一个 `UserService.java` 接口和一个 `UserController.java` 控制器。示例代码如下:
```java
package com.example.demo.service;
import com.example.demo.entity.User;
import java.util.List;
public interface UserService {
User getUserById(int id);
List<User> getAllUsers();
}
```
```java
package com.example.demo.service.impl;
import com.example.demo.dao.UserDao;
import com.example.demo.entity.User;
import com.example.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserDao userDao;
@Override
public User getUserById(int id) {
return userDao.getUserById(id);
}
@Override
public List<User> getAllUsers() {
return userDao.getAllUsers();
}
}
```
```java
package com.example.demo.controller;
import com.example.demo.entity.User;
import com.example.demo.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public User getUserById(@PathVariable int id) {
return userService.getUserById(id);
}
@GetMapping("/users")
public List<User> getAllUsers() {
return userService.getAllUsers();
}
}
```
6. 运行应用程序
在终端中输入以下命令:
```
mvn spring-boot:run
```
然后在浏览器中访问以下 URL:
```
http://localhost:8080/users/1
http://localhost:8080/users
```
就可以看到查询结果了。
阅读全文
相关推荐
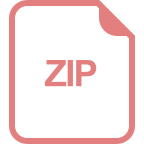

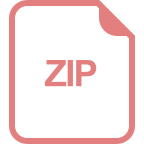
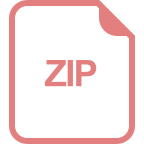
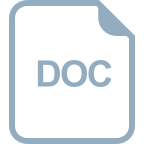
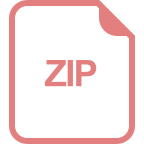
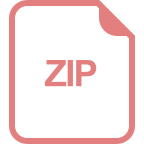
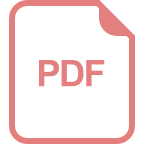
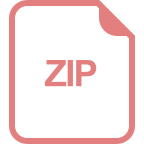
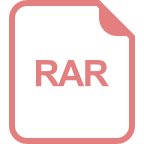
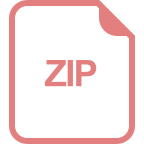
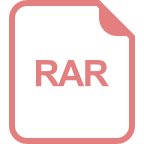
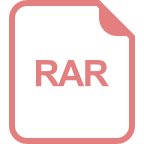
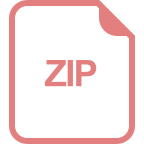
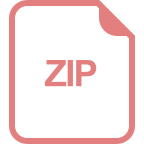
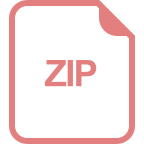
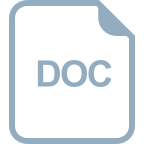