python贪吃蛇代码
时间: 2023-07-09 10:46:31 浏览: 69
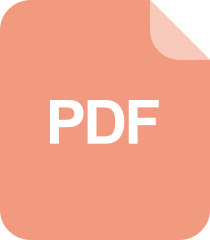
python贪吃蛇游戏代码

以下是一个简单的Python贪吃蛇游戏代码:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 定义窗口大小
SCREEN_WIDTH = 600
SCREEN_HEIGHT = 600
# 定义贪吃蛇速度
SNAKE_SPEED = 15
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
# 创建窗口
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("Snake Game")
# 定义贪吃蛇初始位置和长度
snake_pos = [300, 300]
snake_body = [[300, 300], [290, 300], [280, 300]]
# 定义食物初始位置
food_pos = [random.randrange(1, (SCREEN_WIDTH//10)) * 10,
random.randrange(1, (SCREEN_HEIGHT//10)) * 10]
food_spawn = True
# 定义运动方向
direction = "RIGHT"
change_to = direction
# 定义分数
score = 0
# 定义字体
font = pygame.font.SysFont('arial', 25)
# 定义游戏结束函数
def game_over():
font1 = pygame.font.SysFont('arial', 50)
line1 = font1.render("Game Over!", True, RED)
line2 = font1.render("Your final score is: " + str(score), True, WHITE)
screen.blit(line1, (SCREEN_WIDTH/3, SCREEN_HEIGHT/3))
screen.blit(line2, (SCREEN_WIDTH/3, SCREEN_HEIGHT/2))
pygame.display.update()
pygame.time.wait(3000)
pygame.quit()
quit()
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
change_to = "LEFT"
elif event.key == pygame.K_RIGHT:
change_to = "RIGHT"
elif event.key == pygame.K_UP:
change_to = "UP"
elif event.key == pygame.K_DOWN:
change_to = "DOWN"
elif event.key == pygame.K_ESCAPE:
pygame.quit()
quit()
# 判断运动方向是否合法
if change_to == "LEFT" and direction != "RIGHT":
direction = "LEFT"
elif change_to == "RIGHT" and direction != "LEFT":
direction = "RIGHT"
elif change_to == "UP" and direction != "DOWN":
direction = "UP"
elif change_to == "DOWN" and direction != "UP":
direction = "DOWN"
# 根据方向更新贪吃蛇位置
if direction == "LEFT":
snake_pos[0] -= 10
elif direction == "RIGHT":
snake_pos[0] += 10
elif direction == "UP":
snake_pos[1] -= 10
elif direction == "DOWN":
snake_pos[1] += 10
# 更新贪吃蛇身体
snake_body.insert(0, list(snake_pos))
if snake_pos == food_pos:
food_spawn = False
score += 10
else:
snake_body.pop()
# 重新生成食物
if not food_spawn:
food_pos = [random.randrange(1, (SCREEN_WIDTH//10)) * 10,
random.randrange(1, (SCREEN_HEIGHT//10)) * 10]
food_spawn = True
# 绘制背景
screen.fill(BLACK)
# 绘制贪吃蛇身体
for pos in snake_body:
pygame.draw.rect(screen, WHITE, pygame.Rect(
pos[0], pos[1], 10, 10))
# 绘制食物
pygame.draw.rect(screen, RED, pygame.Rect(
food_pos[0], food_pos[1], 10, 10))
# 绘制分数
score_font = font.render("Score: " + str(score), True, WHITE)
screen.blit(score_font, (0, 0))
# 判断贪吃蛇是否撞墙
if snake_pos[0] < 0 or snake_pos[0] > SCREEN_WIDTH-10:
game_over()
elif snake_pos[1] < 0 or snake_pos[1] > SCREEN_HEIGHT-10:
game_over()
# 判断贪吃蛇是否撞到自己
for block in snake_body[1:]:
if snake_pos == block:
game_over()
# 更新屏幕
pygame.display.update()
# 控制游戏速度
pygame.time.Clock().tick(SNAKE_SPEED)
```
这个代码实现了一个基本的贪吃蛇游戏,运行后可以使用方向键控制贪吃蛇的运动方向,吃到食物后会增加分数并且重新生成食物。当贪吃蛇撞墙或者撞到自己时游戏结束,并且显示最终得分。
阅读全文
相关推荐

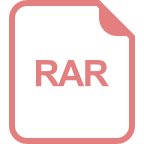
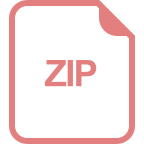