能否帮助我用哈希表编写一个简单的学生管理系统,要求能够从键盘输入学号和姓名,人数限定五人
时间: 2024-01-26 09:04:43 浏览: 84
好的,这里提供一个C++版本的简单学生管理系统,使用哈希表实现。代码如下:
```c++
#include <iostream>
#include <string>
using namespace std;
const int MAX_STUDENTS = 5; // 学生人数上限
const int TABLE_SIZE = 10; // 哈希表大小
// 学生类
class Student {
private:
int id;
string name;
public:
Student() {}
Student(int id, string name) {
this->id = id;
this->name = name;
}
int getId() { return id; }
string getName() { return name; }
};
// 哈希表节点类
class Node {
private:
int key;
Student value;
public:
Node() {}
Node(int key, Student value) {
this->key = key;
this->value = value;
}
int getKey() { return key; }
Student getValue() { return value; }
};
// 哈希表类
class HashMap {
private:
Node* table[TABLE_SIZE];
public:
HashMap() {
for (int i = 0; i < TABLE_SIZE; i++) {
table[i] = nullptr;
}
}
~HashMap() {
for (int i = 0; i < TABLE_SIZE; i++) {
delete table[i];
}
}
// 哈希函数
int hash(int key) {
return key % TABLE_SIZE;
}
// 插入节点
void put(int key, Student value) {
int index = hash(key);
while (table[index] != nullptr && table[index]->getKey() != key) {
index = (index + 1) % TABLE_SIZE;
}
if (table[index] != nullptr) {
delete table[index];
}
table[index] = new Node(key, value);
}
// 查找节点
Student get(int key) {
int index = hash(key);
while (table[index] != nullptr && table[index]->getKey() != key) {
index = (index + 1) % TABLE_SIZE;
}
if (table[index] == nullptr) {
return Student(-1, "");
}
else {
return table[index]->getValue();
}
}
};
int main() {
HashMap map;
int count = 0;
while (count < MAX_STUDENTS) {
int id;
string name;
cout << "请输入第" << count + 1 << "个学生的学号和姓名:" << endl;
cin >> id >> name;
map.put(id, Student(id, name));
count++;
}
cout << "学生信息如下:" << endl;
for (int i = 0; i < TABLE_SIZE; i++) {
Node* node = map.table[i];
if (node != nullptr) {
Student student = node->getValue();
cout << student.getId() << "\t" << student.getName() << endl;
}
}
return 0;
}
```
在这个实现中,我们使用了一个 `HashMap` 类来表示哈希表。节点类 `Node` 中存储了键值对,键是学生的学号,值是学生对象。哈希函数使用简单的取模运算,冲突处理采用了线性探测法。在 `main` 函数中,我们从键盘输入学号和姓名,插入到哈希表中。最后遍历哈希表,输出学生信息。
阅读全文
相关推荐
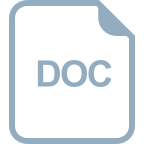
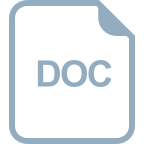
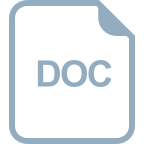
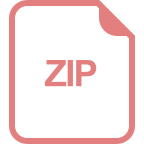


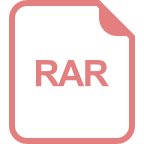
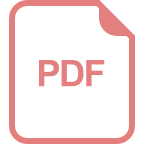
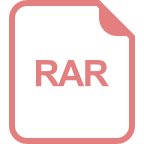
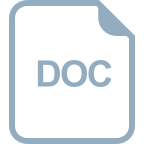







