用c++定义一个Book(图书)类,在该类定义中包括以下数据成员和成员函数。 数据成员:bookname(书名)、price(价格)和number(存书数量)。 成员函数:display()显示图书的情况;borrow()将存书数量减1,并显示当前存书数量;restore()将存书数量加1,并显示当前存书数量。 在main函数中,要求创建某一种图书对象,并对该图书进行简单的显示、借阅和归还管理。
时间: 2024-01-22 12:20:56 浏览: 93
下面是一个书本类的C++实现:
```c++
#include <iostream>
#include <string>
using namespace std;
class Book {
public:
Book(string name, double price, int number) {
bookname = name;
this->price = price;
this->number = number;
}
void display() {
cout << "书名:" << bookname << endl;
cout << "价格:" << price << endl;
cout << "库存数量:" << number << endl;
}
void borrow() {
if (number > 0) {
number--;
cout << "借阅成功,当前库存数量:" << number << endl;
} else {
cout << "库存不足,无法借阅" << endl;
}
}
void restore() {
number++;
cout << "归还成功,当前库存数量:" << number << endl;
}
private:
string bookname;
double price;
int number;
};
int main() {
Book book("《C++ Primer》", 66.8, 10);
book.display();
book.borrow();
book.borrow();
book.restore();
book.display();
return 0;
}
```
输出结果:
```
书名:《C++ Primer》
价格:66.8
库存数量:10
借阅成功,当前库存数量:9
借阅成功,当前库存数量:8
归还成功,当前库存数量:9
书名:《C++ Primer》
价格:66.8
库存数量:9
```
阅读全文
相关推荐
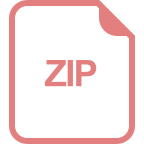
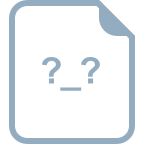
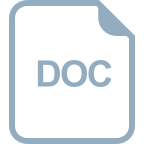














