#include<iostream> using namespace std; class Book { private: string bookname; string authtors; string publishing_house; int pages; double price; public: Book() { bookname = ""; authtors = ""; publishing_house = ""; pages = 0; price = 0; } Book(string bk, string auth, string pub, int pag,double pri) { bookname = bk; authtors = auth; publishing_house = pub; pages = pag; price = pri; } ~Book(){} string getBookname() const { return bookname; } void setBookname(char*name){ bookname=name; } string getAuthtors() const { return authtors; } void setAuthtors(char* auth) { authtors = auth; } string getPublishing_house() const { return publishing_house; } void setPublishing_house(char* pub) { publishing_house = pub; } int getPages() const { return pages; } void setPages(int pag) { pages = pag; } double getPrice() const { return price; } void setPrice(double pri) { price = pri; } }; int main() { Book book1("《C++程序设计》", "谭浩强编著", 485, "清华大学出版社", 36.00); Book book2("《吹牛大王历险记》", "拉斯伯等编著", 149, "天津人民出版社", 12.80); cout << "书名:" << book1.getBookname() << endl; cout << "作者:" << book1.getAuthtors() << endl; cout << "页数:" << book1.getPages() <<"页"<< endl; cout << "出版社:" << book1.getPublishing_house() << endl; cout << "价格:" << book1.getPrice() <<"元"<< endl; cout << endl; cout << "书名:" << book2.getBookname() << endl; cout << "作者:" << book2.getAuthtors() << endl; cout << "页数:" << book2.getPages() << "页" << endl; cout << "出版社:" << book2.getPublishing_house() << endl; cout << "价格:" << book2.getPrice() << "元" << endl; return 0; }这个代码如何修改
时间: 2023-06-26 14:05:05 浏览: 73
这段代码有一些问题,比如在类的成员函数中使用了字符指针而不是字符串,还有构造函数和成员函数的参数顺序不一致。下面是修改后的代码:
```
#include <iostream>
#include <string>
using namespace std;
class Book {
private:
string bookname;
string authtors;
string publishing_house;
int pages;
double price;
public:
Book() {
bookname = "";
authtors = "";
publishing_house = "";
pages = 0;
price = 0;
}
Book(string bk, string auth, int pag, string pub, double pri) {
bookname = bk;
authtors = auth;
pages = pag;
publishing_house = pub;
price = pri;
}
~Book() {}
string getBookname() const {
return bookname;
}
void setBookname(string name) {
bookname = name;
}
string getAuthtors() const {
return authtors;
}
void setAuthtors(string auth) {
authtors = auth;
}
string getPublishing_house() const {
return publishing_house;
}
void setPublishing_house(string pub) {
publishing_house = pub;
}
int getPages() const {
return pages;
}
void setPages(int pag) {
pages = pag;
}
double getPrice() const {
return price;
}
void setPrice(double pri) {
price = pri;
}
};
int main() {
Book book1("《C++程序设计》", "谭浩强编著", 485, "清华大学出版社", 36.00);
Book book2("《吹牛大王历险记》", "拉斯伯等编著", 149, "天津人民出版社", 12.80);
cout << "书名:" << book1.getBookname() << endl;
cout << "作者:" << book1.getAuthtors() << endl;
cout << "页数:" << book1.getPages() <<"页"<< endl;
cout << "出版社:" << book1.getPublishing_house() << endl;
cout << "价格:" << book1.getPrice() <<"元"<< endl;
cout << endl;
cout << "书名:" << book2.getBookname() << endl;
cout << "作者:" << book2.getAuthtors() << endl;
cout << "页数:" << book2.getPages() << "页" << endl;
cout << "出版社:" << book2.getPublishing_house() << endl;
cout << "价格:" << book2.getPrice() << "元" << endl;
return 0;
}
```
这个修改后的代码使用了字符串来代替字符指针,并将参数顺序保持一致。这样代码更加清晰易读,并且更符合 C++ 的编程风格。
相关推荐
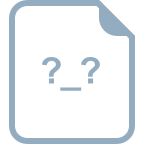
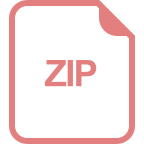
















