请用C++实现一个 Cuckoo Hash,一旦判断发生循环路径之后,进行 Rehash 操作,选择新的 Hash 函数,然后重新构造整个哈希 表。
时间: 2023-06-24 16:07:07 浏览: 196
Cuckoo Hash 是一种高效的哈希表实现,可以解决哈希冲突问题。当出现哈希冲突时,Cuckoo Hash 采用另一个哈希函数将冲突的元素插入到另一个哈希桶中,而不是在当前哈希桶中进行线性探测或者开放地址探测。
下面是一个简单的 C++ 实现:
```c++
#include <iostream>
#include <vector>
#include <functional>
template<typename T>
class CuckooHash {
private:
std::vector<T> table1;
std::vector<T> table2;
std::vector<bool> used1;
std::vector<bool> used2;
std::hash<T> hashFunc;
std::hash<T> hashFunc2;
size_t capacity;
size_t size;
public:
CuckooHash(size_t cap) : capacity(cap), size(0) {
table1.resize(capacity);
table2.resize(capacity);
used1.resize(capacity, false);
used2.resize(capacity, false);
}
void insert(T item) {
while (true) {
if (size == capacity) {
rehash();
}
size_t index1 = hashFunc(item) % capacity;
if (used1[index1]) {
T temp = table1[index1];
table1[index1] = item;
item = temp;
} else {
table1[index1] = item;
used1[index1] = true;
size++;
return;
}
size_t index2 = hashFunc2(item) % capacity;
if (used2[index2]) {
T temp = table2[index2];
table2[index2] = item;
item = temp;
} else {
table2[index2] = item;
used2[index2] = true;
size++;
return;
}
}
}
bool remove(T item) {
size_t index1 = hashFunc(item) % capacity;
if (used1[index1] && table1[index1] == item) {
used1[index1] = false;
size--;
return true;
}
size_t index2 = hashFunc2(item) % capacity;
if (used2[index2] && table2[index2] == item) {
used2[index2] = false;
size--;
return true;
}
return false;
}
bool contains(T item) {
size_t index1 = hashFunc(item) % capacity;
if (used1[index1] && table1[index1] == item) {
return true;
}
size_t index2 = hashFunc2(item) % capacity;
if (used2[index2] && table2[index2] == item) {
return true;
}
return false;
}
void rehash() {
capacity *= 2;
size = 0;
table1.clear();
table2.clear();
used1.clear();
used2.clear();
table1.resize(capacity);
table2.resize(capacity);
used1.resize(capacity, false);
used2.resize(capacity, false);
for (size_t i = 0; i < capacity / 2; i++) {
if (used1[i]) {
insert(table1[i]);
}
if (used2[i]) {
insert(table2[i]);
}
}
}
};
```
在上面的实现中,我们使用了两个哈希表 `table1` 和 `table2`,以及两个对应的布尔数组 `used1` 和 `used2` 来存储元素和判断元素是否被占用。在插入元素时,我们先使用第一个哈希函数计算出元素的桶索引,如果该桶已经被占用,我们就将其中的元素拿出来,将当前元素插入到该桶中,然后将拿出来的元素作为当前元素,再使用第二个哈希函数计算出另一个桶索引,重复上述过程。如果在插入元素的过程中发现循环路径,我们就进行 Rehash 操作,选择新的 Hash 函数,然后重新构造整个哈希表。
参考资料:
- [Cuckoo Hashing](https://www.cs.cmu.edu/~avrim/451f11/lectures/lect1006.pdf)
- [Cuckoo Hashing: Another Hash Table Scheme](https://www.seas.harvard.edu/courses/cs165/papers/cuckoo-conext2014.pdf)
阅读全文
相关推荐
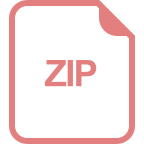
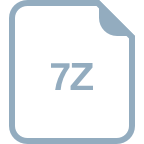
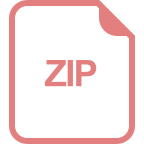
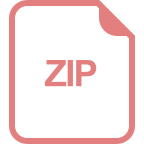
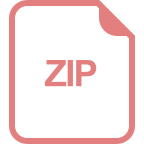
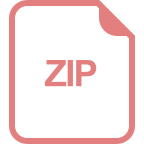