python swagger
时间: 2023-12-05 09:41:33 浏览: 150
Swagger是一种用于描述、生产、消费和可视化RESTful Web服务的开源软件。它包括一个规范和一组工具,可以帮助开发人员设计、构建、文档化和测试RESTful Web服务。Swagger规范定义了一种标准的、语言无关的接口描述格式,可以用于描述RESTful Web服务的API。Swagger工具集包括Swagger UI、Swagger Editor和Swagger Codegen等工具,可以帮助开发人员可视化RESTful Web服务的API、编辑Swagger规范、生成客户端和服务器端代码等。
以下是使用Python编写RESTful Web服务并使用Swagger进行API文档化的步骤:
1.安装Flask和Flask-RESTful库
```shell
pip install flask
pip install flask-restful
```
2.编写RESTful Web服务代码
```python
from flask import Flask
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
class HelloWorld(Resource):
def get(self):
return {'hello': 'world'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
```
3.安装Flasgger库
```shell
pip install flasgger
```
4.使用Flasgger进行API文档化
```python
from flask import Flask
from flask_restful import Resource, Api
from flasgger import Swagger
app = Flask(__name__)
api = Api(app)
swagger = Swagger(app)
class HelloWorld(Resource):
def get(self):
"""
This is an example endpoint that returns 'hello world'.
---
responses:
200:
description: A string that says 'hello world'.
"""
return {'hello': 'world'}
api.add_resource(HelloWorld, '/')
if __name__ == '__main__':
app.run(debug=True)
```
在浏览器中访问http://localhost:5000/apidocs,即可看到使用Swagger生成的API文档。
阅读全文
相关推荐
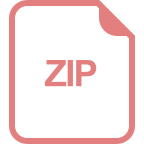
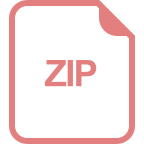



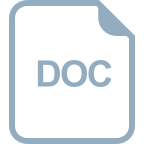







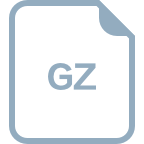
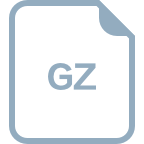
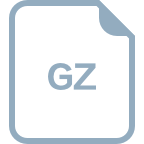