stc89c52rc单片机基于c语言实现系统运行时可以通过串口助手下发的指令进行时间的设置:下发的指令格式为:dataset ××: ××: ××(设置日期);timeset ××: ××: ××(设置时间)。
时间: 2024-05-10 14:15:22 浏览: 3
好的,你需要编写以下功能:
1. 串口初始化函数,配置波特率、数据位、停止位等参数。
2. 日期时间设置函数,解析下发的指令并设置系统时间。
3. 串口接收函数,接收下发的指令。
4. 主函数中需要循环监听串口接收到的指令,并调用日期时间设置函数。
下面是代码示例:
```c
#include <reg52.h>
#include <stdio.h>
// 定义串口参数
#define BAUDRATE 9600
#define DIVIDER (65536 - FOSC / 32 / BAUDRATE)
// 定义系统时间结构体
typedef struct {
unsigned char year; // 年份
unsigned char month; // 月份
unsigned char day; // 日
unsigned char hour; // 小时
unsigned char minute; // 分钟
unsigned char second; // 秒钟
} datetime_t;
// 定义全局变量
datetime_t datetime;
// 串口初始化函数
void uart_init() {
SCON = 0x50; // 配置为8位数据位,无校验位,1位停止位
TMOD &= 0x0F; // 清空计数器1模式设置
TMOD |= 0x20; // 设置计数器1为模式2:自动重载
TH1 = DIVIDER / 256; // 设置计数器1重载值
TL1 = DIVIDER % 256;
TR1 = 1; // 启动计数器1
ES = 1; // 允许串口中断
EA = 1; // 允许总中断
}
// 日期时间设置函数
void datetime_set(char* cmd) {
unsigned char year, month, day, hour, minute, second;
// 解析指令
sscanf(cmd, "dataset %hhu:%hhu:%hhu", &year, &month, &day);
sscanf(cmd, "timeset %hhu:%hhu:%hhu", &hour, &minute, &second);
// 设置系统时间
datetime.year = year;
datetime.month = month;
datetime.day = day;
datetime.hour = hour;
datetime.minute = minute;
datetime.second = second;
}
// 串口接收函数
void uart_isr() interrupt 4 {
unsigned char c;
if (RI) { // 接收中断
c = SBUF; // 读取接收到的数据
RI = 0; // 清除接收中断标志
// 处理接收到的指令
static char cmd[32];
static int pos = 0;
if (c == '\r' || c == '\n') { // 遇到回车或换行符
cmd[pos] = '\0'; // 添加字符串结束符
datetime_set(cmd); // 执行指令
pos = 0; // 重置指令缓存
} else {
cmd[pos++] = c; // 缓存指令
}
}
}
// 主函数
int main() {
uart_init(); // 初始化串口
while (1) {
// 主循环中无需执行其他操作,只需要等待串口接收中断即可
}
return 0;
}
```
在实际使用中,你需要根据自己的需求进行调整和优化。
相关推荐
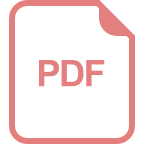
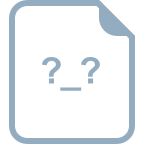














