python连接plc代码
时间: 2024-03-12 16:42:15 浏览: 177
在Python中连接PLC的代码可以使用第三方库来实,最常用的是pycomm库。下面是一个简单的示例代码,演示了如何使用pycomm库连接PLC:
```python
from pycomm.ab_comm.clx import Driver as ClxDriver
# 创建PLC连接对象
plc = ClxDriver()
# 连接PLC
plc.open('192.168.1.1') # 替换为你的PLC IP地址
# 读取PLC的数据
data = plc.read('tag_name') # 替换为你要读取的标签名
# 写入数据到PLC
plc.write('tag_name', 123) # 替换为你要写入的标签名和值
# 关闭PLC连接
plc.close()
```
请注意,上述代码中的IP地址和标签名需要根据你的实际情况进行替换。另外,pycomm库还支持其他类型的PLC连接,如ControlLogix、CompactLogix等,你可以根据自己的PLC型号选择相应的驱动。
相关问题
Python 三菱PLC连接 代码
Python 三菱PLC连接的代码需要使用到第三方库 pymodbus,你可以通过pip安装该库。以下是一个简单的Python代码示例,用于连接到三菱PLC并读取寄存器的值:
```
from pymodbus.client.sync import ModbusTcpClient
# 创建一个连接
client = ModbusTcpClient('192.168.1.100', port=502)
# 建立连接
client.connect()
# 读取寄存器的值
result = client.read_holding_registers(address=0, count=10, unit=1)
# 关闭连接
client.close()
# 打印结果
print(result.registers)
```
上述代码中,我们通过 ModbusTcpClient 创建了一个连接,并使用 connect() 方法建立了连接。然后,我们使用 read_holding_registers() 方法读取了从地址0开始的10个寄存器的值,并将结果存储在 result 变量中。最后,我们使用 close() 方法关闭连接。
Python连接plc如何封装,做到掉线重启线程
在Python中,连接PLC并实现断线后自动重启线程,通常会使用`threading`模块来处理线程管理和异常处理。首先,你需要安装合适的库来连接PLC,比如`modbus-tcp`、`pymodbus`等。
下面是一个简单的示例,展示了如何封装这个功能:
```python
import threading
import time
from pymodbus.client.sync import ModbusTcpClient
class PLCConnectionThread(threading.Thread):
def __init__(self, plc_ip, plc_port):
super().__init__()
self.plc = ModbusTcpClient(plc_ip, plc_port)
self.is_alive = True
self.daemon = True # 设置线程为守护线程,在主线程退出时自动结束
def run(self):
while self.is_alive:
try:
# 这里放置读取或写入PLC的操作
response = self.plc.read_holding_registers(0, 1) # 示例代码
if response.isError():
print("PLC connection lost, retrying...")
else:
process_response(response.registers)
# 添加定时检查连接是否存活的机制
time.sleep(5) # 每隔5秒检查一次
except Exception as e:
print(f"Error occurred: {str(e)}")
self.restart_connection()
def restart_connection(self):
self.plc.close()
# 等待一段时间后再尝试重新连接
time.sleep(10)
self.plc = ModbusTcpClient(self.plc_ip, self.plc_port)
# 如果连接成功,继续运行循环
# 使用示例
plc_thread = PLCConnectionThread('your_plc_ip', 502)
plc_thread.start()
```
在这个示例中,`PLCConnectionThread`类负责维持PLC的连接,并在检测到网络问题时主动关闭连接并在一定时间后重新建立。你可以根据实际需求调整连接检查的频率和其他细节。
阅读全文
相关推荐
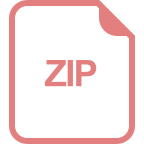
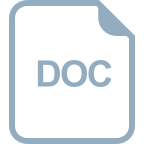












