用C语言进行编程:对句子中的各个单词按照字母'o'出现的次数排序(由多到少) 字母'o'数量相同的单词从小到大排列; 将排序好的单词输出,每行一个。
时间: 2024-02-06 16:02:48 浏览: 122
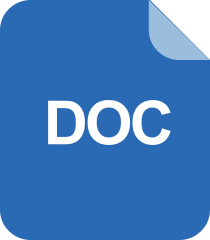
C语言编程题精选 C语言编程题精选
好的,这是一个比较有挑战性的问题,需要进行字符串处理和排序。我来给你提供一个思路:
1. 定义一个结构体,包含两个字段:单词和'o'的数量;
2. 使用scanf()函数读入句子,使用strtok()函数将句子分割成单词;
3. 对于每个单词,遍历每个字符,记录字母'o'的数量;
4. 将单词和'o'的数量存入结构体数组中;
5. 使用qsort()函数对结构体数组进行排序,先按照'o'的数量由多到少排序,如果'o'的数量相同,则按照单词的字典序进行排序;
6. 输出排序好的单词。
以下是参考代码,仅供参考:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAX_WORD_LEN 50
#define MAX_WORDS_NUM 100
struct Word {
char str[MAX_WORD_LEN];
int o_count;
};
int cmp(const void* a, const void* b) {
const struct Word* word_a = a;
const struct Word* word_b = b;
if (word_a->o_count != word_b->o_count) {
return word_b->o_count - word_a->o_count;
} else {
return strcmp(word_a->str, word_b->str);
}
}
int main() {
char sentence[MAX_WORDS_NUM * MAX_WORD_LEN];
struct Word words[MAX_WORDS_NUM];
int word_num = 0;
// 读入句子
printf("请输入句子:\n");
fgets(sentence, MAX_WORDS_NUM * MAX_WORD_LEN, stdin);
// 分割单词
char* word = strtok(sentence, " ,.?!\"");
while (word != NULL) {
// 统计'o'的数量
int o_count = 0;
for (int i = 0; i < strlen(word); i++) {
if (word[i] == 'o' || word[i] == 'O') {
o_count++;
}
}
// 存入结构体数组中
strcpy(words[word_num].str, word);
words[word_num].o_count = o_count;
word_num++;
word = strtok(NULL, " ,.?!\"");
}
// 排序并输出单词
qsort(words, word_num, sizeof(struct Word), cmp);
printf("按照'o'的数量排序后的单词:\n");
for (int i = 0; i < word_num; i++) {
printf("%s\n", words[i].str);
}
return 0;
}
```
阅读全文
相关推荐
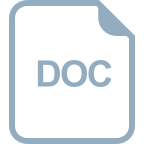
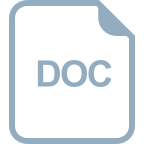
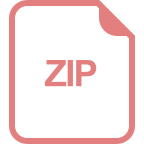
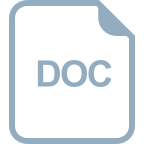
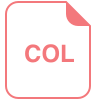




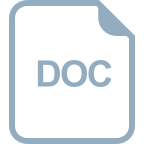
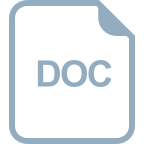
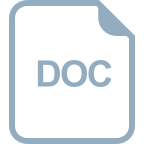
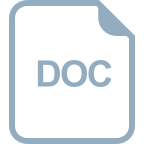
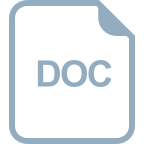
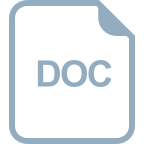
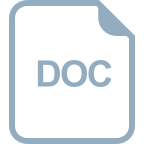