C语言下,输入一个单词,找出单词中出现次数最多的字母的出现次数
时间: 2024-01-22 21:14:30 浏览: 215
以下是C语言的代码,可以输入一个单词,找出单词中出现次数最多的字母的出现次数:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char word[100];
int count[26] = {0}, max_count = 0;
printf("请输入一个单词:");
scanf("%s", word);
int length = strlen(word);
for (int i = 0; i < length; i++) {
count[word[i] - 'a']++;
}
for (int i = 0; i < 26; i++) {
if (count[i] > max_count) {
max_count = count[i];
}
}
printf("出现次数最多的字母出现了 %d 次。\n", max_count);
return 0;
}
```
相关问题
c语言:统计一个英文的文本文件中出现次数最多的前十个单词(1)规定单词的含义为连续的字母构成的字符串,字母以外的其他符号和空白符号都视为单词之间的分隔符。(2)输出出现次数最多的前十个单词及出现次数。仅大小写不同的单词视为同一单词。
好的,这是一个比较典型的统计单词出现频率的问题。我们可以使用哈希表来实现。具体步骤如下:
1. 读入文本文件,将单词逐个提取出来并转换为小写字母。
2. 对于每个单词,将其作为键值,将对应的哈希表中的值加1。
3. 遍历哈希表,找到出现次数最多的前十个单词。
下面是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#include <string.h>
#define MAX_WORD_LEN 50
#define HASH_SIZE 1000000
typedef struct Node {
char* key;
int val;
struct Node* next;
} Node;
Node* hash_table[HASH_SIZE];
// 哈希函数
unsigned long hash(char* str) {
unsigned long hash = 5381;
int c;
while ((c = *str++))
hash = ((hash << 5) + hash) + c;
return hash % HASH_SIZE;
}
// 向哈希表中插入键值对
void insert(char* key, int val) {
unsigned long h = hash(key);
Node* p = hash_table[h];
while (p != NULL) {
if (strcmp(p->key, key) == 0) {
p->val += val;
return;
}
p = p->next;
}
Node* node = (Node*)malloc(sizeof(Node));
node->key = strdup(key);
node->val = val;
node->next = hash_table[h];
hash_table[h] = node;
}
// 统计文本文件中出现次数最多的前十个单词
void count_words(FILE* fp) {
char word[MAX_WORD_LEN];
int ch, pos = 0;
while ((ch = fgetc(fp)) != EOF) {
if (isalpha(ch)) {
word[pos++] = tolower(ch);
} else if (pos > 0) {
word[pos] = '\0';
insert(word, 1);
pos = 0;
}
}
if (pos > 0) {
word[pos] = '\0';
insert(word, 1);
}
// 找出出现次数最多的前十个单词
Node* top_ten[10] = { NULL };
Node* p;
for (int i = 0; i < HASH_SIZE; i++) {
p = hash_table[i];
while (p != NULL) {
for (int j = 0; j < 10; j++) {
if (top_ten[j] == NULL || p->val > top_ten[j]->val) {
for (int k = 9; k > j; k--) {
top_ten[k] = top_ten[k - 1];
}
top_ten[j] = p;
break;
} else if (strcmp(p->key, top_ten[j]->key) == 0) {
top_ten[j] = p;
break;
}
}
p = p->next;
}
}
// 输出结果
for (int i = 0; i < 10; i++) {
if (top_ten[i] != NULL) {
printf("%-20s %d\n", top_ten[i]->key, top_ten[i]->val);
}
}
}
int main() {
memset(hash_table, 0, sizeof(hash_table));
FILE* fp = fopen("test.txt", "r");
if (fp == NULL) {
printf("Failed to open file.\n");
return 1;
}
count_words(fp);
fclose(fp);
return 0;
}
```
在这个实现中,我们使用了一个数组 `hash_table` 来表示哈希表。哈希表的每个元素是一个指向链表头的指针,链表中存储了键值对。
我们使用了一个字符串哈希函数,将字符串转换为一个 unsigned long 值。这个哈希函数的实现来自于 DJB 算法。
在 `count_words` 函数中,我们逐个读入文本文件中的字符,并将连续的字母构成的字符串转换为小写字母后插入哈希表中。在插入时,如果键值已经存在,则将对应的值加1。
最后,我们遍历哈希表,找到出现次数最多的前十个单词。我们使用一个数组 `top_ten` 来存储前十个单词的指针。在遍历哈希表时,对于每个键值对,我们将其与 `top_ten` 中的元素比较,将其插入到合适的位置。
最后,我们输出前十个单词及其出现次数。注意,在输出时,我们使用了格式化字符串 `%-20s %d\n`,这是为了让输出对齐。
,给了一个单词后,帮助他找到出现最多的字母和这 个字母出现的次数用C语言写一段代码
### 回答1:
嗯,我可以给你一个参考:#include <stdio.h>int main()
{
char str[100];
int i, j, count;
char maxChar;
int maxCount = 0;
printf("请输入一个单词:");
scanf("%s", str);
for (i = 0; str[i] != '\0'; ++i)
{
count = 1;
for (j = i + 1; str[j] != '\0'; ++j)
{
if (str[i] == str[j])
++count;
}
if (count > maxCount)
{
maxCount = count;
maxChar = str[i];
}
}
printf("字母 %c 出现最多,次数为 %d。", maxChar, maxCount);
return 0;
}
### 回答2:
#include <stdio.h>
#include <string.h>
void findMostFrequentChar(char word[]) {
int count[26] = {0};
int maxCount = 0;
char maxChar;
for (int i = 0; i < strlen(word); i++) {
if (word[i] >= 'a' && word[i] <= 'z') {
count[word[i] - 'a']++;
}
else if (word[i] >= 'A' && word[i] <= 'Z') {
count[word[i] - 'A']++;
}
}
for (int i = 0; i < 26; i++) {
if (count[i] > maxCount) {
maxCount = count[i];
maxChar = 'a' + i;
}
}
printf("出现最多的字母是%c,出现的次数是%d\n", maxChar, maxCount);
}
int main() {
char word[100];
printf("请输入单词:");
scanf("%s", word);
findMostFrequentChar(word);
return 0;
}
### 回答3:
下面是一个使用C语言编写的代码,该代码能够接收一个单词作为输入,并找出在单词中出现最多的字母以及该字母出现的次数。
```c
#include <stdio.h>
#include <string.h>
#define MAX_SIZE 100
int main() {
char word[MAX_SIZE];
char maxChar;
int maxCount = 0;
int i, j, count;
printf("请输入一个单词:");
scanf("%s", word);
// 遍历单词中的每个字母
for (i = 0; word[i] != '\0'; i++) {
count = 0;
// 检查当前字母在单词中出现的次数
for (j = 0; word[j] != '\0'; j++) {
if (word[i] == word[j])
count++;
}
// 更新最大次数和字母
if (count > maxCount) {
maxCount = count;
maxChar = word[i];
}
}
printf("出现最多的字母是 %c,出现次数为 %d\n", maxChar, maxCount);
return 0;
}
```
运行该代码,输入一个单词后,代码将会输出该单词中出现最多的字母以及该字母出现的次数。
阅读全文
相关推荐
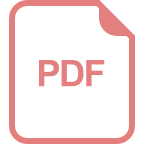
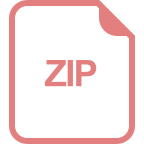












