图的创建以及深度优先遍历和广度优先遍历C语言代码实现
时间: 2023-10-04 22:08:52 浏览: 121
以下是图的创建以及深度优先遍历和广度优先遍历的C语言代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 50
typedef struct graphNode {
int vertex;
struct graphNode* next;
} graphNode;
void addEdge(graphNode** adjList, int u, int v) {
graphNode* newNode = (graphNode*)malloc(sizeof(graphNode));
newNode->vertex = v;
newNode->next = adjList[u];
adjList[u] = newNode;
}
int visited[MAX_VERTICES];
void DFS(graphNode** adjList, int vertex) {
visited[vertex] = 1;
printf("%d ", vertex);
graphNode* temp = adjList[vertex];
while (temp) {
int adjVertex = temp->vertex;
if (!visited[adjVertex]) {
DFS(adjList, adjVertex);
}
temp = temp->next;
}
}
void BFS(graphNode** adjList, int vertex, int numVertices) {
int queue[MAX_VERTICES];
int front = -1, rear = -1;
queue[++rear] = vertex;
visited[vertex] = 1;
while (front != rear) {
int currVertex = queue[++front];
printf("%d ", currVertex);
graphNode* temp = adjList[currVertex];
while (temp) {
int adjVertex = temp->vertex;
if (!visited[adjVertex]) {
visited[adjVertex] = 1;
queue[++rear] = adjVertex;
}
temp = temp->next;
}
}
}
int main() {
int numVertices, numEdges, i, u, v;
printf("Enter the number of vertices: ");
scanf("%d", &numVertices);
graphNode* adjList[numVertices];
for (i = 0; i < numVertices; i++) {
adjList[i] = NULL;
}
printf("Enter the number of edges: ");
scanf("%d", &numEdges);
for (i = 0; i < numEdges; i++) {
printf("Enter edge %d (u, v): ", i + 1);
scanf("%d%d", &u, &v);
addEdge(adjList, u, v);
addEdge(adjList, v, u);
}
printf("Adjacency List:\n");
for (i = 0; i < numVertices; i++) {
printf("Vertex %d: ", i);
graphNode* temp = adjList[i];
while (temp) {
printf("%d -> ", temp->vertex);
temp = temp->next;
}
printf("NULL\n");
}
printf("DFS Traversal: ");
for (i = 0; i < numVertices; i++) {
visited[i] = 0;
}
for (i = 0; i < numVertices; i++) {
if (!visited[i]) {
DFS(adjList, i);
}
}
printf("\n");
printf("BFS Traversal: ");
for (i = 0; i < numVertices; i++) {
visited[i] = 0;
}
BFS(adjList, 0, numVertices);
printf("\n");
return 0;
}
```
在此代码实现中,我们首先创建了一个结构体 `graphNode` 用于表示图中的节点,并且定义了一个邻接表 `adjList`,其中每个元素都是一个指向 `graphNode` 的指针。然后我们通过 `addEdge()` 函数来添加边到邻接表中。
接下来,我们实现了深度优先遍历(DFS)和广度优先遍历(BFS)函数。这些函数都需要一个邻接表和一个起始节点作为参数。在 DFS 中,我们首先将当前节点标记为已访问,并输出该节点。然后我们访问该节点的所有未被访问过的邻居节点。在 BFS 中,我们使用一个队列来存储所有已访问的节点,并首先将起始节点入队。然后我们从队列中取出一个节点,输出该节点,并将其所有未被访问过的邻居节点入队。
最后,在 `main()` 函数中,我们首先读入图的节点数和边数。然后我们通过循环来读入每条边,并将其添加到邻接表中。然后我们输出邻接表,并调用 DFS 和 BFS 函数来遍历图。
阅读全文
相关推荐




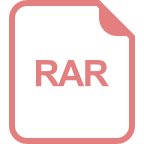













