尾插法建立单链表C语言代码
时间: 2024-09-14 09:17:02 浏览: 50
尾插法是一种常用的单链表的插入方法,它利用了链表尾部的指针(通常是尾节点的next指针)来进行插入操作。这样做的好处是插入操作的时间复杂度可以保持在O(1),即常数时间内完成。下面是一个使用C语言实现尾插法建立单链表的简单例子:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node *next;
} Node;
// 创建一个新节点
Node* createNode(int data) {
Node *newNode = (Node*)malloc(sizeof(Node));
if (newNode) {
newNode->data = data;
newNode->next = NULL;
}
return newNode;
}
// 尾插法建立单链表
Node* createLinkedListByTailInsertion() {
Node *head = NULL, *tail = NULL; // 初始化头指针和尾指针为NULL
int data;
printf("请输入要插入的数据(输入-1结束):\n");
while (1) {
scanf("%d", &data);
if (data == -1) {
break;
}
Node *newNode = createNode(data);
if (tail == NULL) { // 如果链表为空,则新节点既是头节点也是尾节点
head = newNode;
tail = newNode;
} else {
tail->next = newNode; // 将新节点链接到尾节点的后面
tail = newNode; // 更新尾节点为新节点
}
}
return head;
}
// 打印链表
void printList(Node *head) {
Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
// 主函数
int main() {
Node *list = createLinkedListByTailInsertion();
printf("链表元素为:");
printList(list);
// 释放链表内存...
return 0;
}
```
在这个例子中,我们首先定义了链表节点的结构体`Node`,然后通过`createNode`函数创建新的节点。`createLinkedListByTailInsertion`函数利用尾插法创建链表,`printList`函数用于打印链表中的所有元素。注意,在实际使用中,需要在程序结束前释放链表占用的内存,以避免内存泄漏。
阅读全文
相关推荐
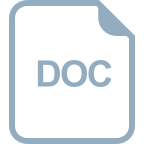
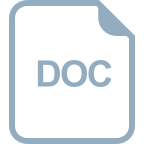
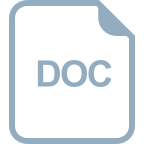


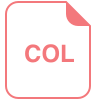










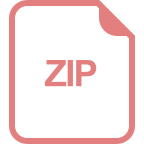

