c语言用尾插法建立一个单链表
时间: 2023-09-22 18:12:06 浏览: 181
尾插法建立单链表的基本思路是:先创建一个头节点,再将新节点依次插入到链表的末尾。
具体实现步骤如下:
1. 定义一个结构体来表示单链表的节点,包括节点值和指向下一个节点的指针。
```
typedef struct Node {
int data;
struct Node* next;
} Node;
```
2. 创建头节点,并初始化链表为空。
```
Node* head = (Node*)malloc(sizeof(Node));
head->next = NULL;
```
3. 循环读入节点值,创建新节点,并将新节点插入到链表的末尾。
```
Node* tail = head; // tail指向链表的末尾节点
int val;
while (scanf("%d", &val) != EOF) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = val;
new_node->next = NULL;
tail->next = new_node; // 将新节点插入到链表的末尾
tail = new_node; // 更新tail指向链表的末尾节点
}
```
完整代码如下:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
int main() {
Node* head = (Node*)malloc(sizeof(Node));
head->next = NULL;
Node* tail = head;
int val;
printf("请输入节点值(以EOF结束):\n");
while (scanf("%d", &val) != EOF) {
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = val;
new_node->next = NULL;
tail->next = new_node;
tail = new_node;
}
printf("链表节点值为:");
Node* p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
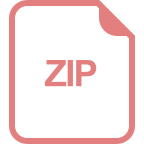















