arduino查找字符串数组中包尾所在的位置,其中字符串数组包含0x00
时间: 2023-06-30 21:11:38 浏览: 143
可以使用 Arduino 的字符串函数库中的 `strchr()` 函数来查找字符串数组中包尾所在的位置,包括字符串数组包含的 `0x00` 字符。`strchr()` 函数的原型如下:
```
char *strchr(const char *str, int c);
```
其中,`str` 参数是要查找的字符串数组,`c` 参数是要查找的字符。函数返回值是指向第一个匹配到的字符的指针,如果没有匹配到则返回 `NULL`。
以下是一个查找包尾所在位置的例子代码,假设字符串数组为 `buf`,包尾字符为 `0x00`:
```cpp
char *tail = strchr(buf, 0x00);
if (tail != NULL) {
int tailIndex = tail - buf; // 计算包尾所在的位置
// 进行后续处理
}
```
在上面的代码中,`tail` 指向包尾字符的指针,如果找到了包尾字符,则计算出它在字符串数组中的位置,并进行后续处理。
相关问题
arduino查找字符串数组中包尾所在的位置,其中字符串数组包含0x00,协议就是上文的协议
好的,下面是一个完整的例子代码,用于查找 Comm_Head 和 Comm_End 数组在一个包含 0x00 字符的字符串数组 buf 中的位置:
```cpp
unsigned char Comm_Head[6] = {0xAA, 0x6F, 0x00, 0x00, 0x00, 0x24};
unsigned char Comm_End[4] = {0xCC, 0x33, 0xC3, 0x3C};
void setup() {
Serial.begin(9600);
while (!Serial) ; // 等待串口连接
}
void loop() {
// 读取串口数据
if (Serial.available()) {
unsigned char buf[64];
int len = Serial.readBytesUntil(0x00, buf, sizeof(buf)); // 读取到包尾为止
if (len > 0) {
// 查找 Comm_Head 和 Comm_End 的位置
char *head = (char *)memmem(buf, len, Comm_Head, sizeof(Comm_Head));
char *end = (char *)memmem(buf, len, Comm_End, sizeof(Comm_End));
if (head != NULL && end != NULL) {
int headIndex = head - (char *)buf;
int endIndex = end - (char *)buf + sizeof(Comm_End) - 1;
// 进行后续处理,比如打印数据
Serial.write(buf + headIndex, endIndex - headIndex + 1);
}
}
}
}
```
上面的代码使用了 Arduino 的串口函数库,每次从串口读取数据并存储到一个大小为 64 的数组 buf 中,使用 `memmem()` 函数查找 Comm_Head 和 Comm_End 在 buf 数组中的位置。`memmem()` 函数可以查找一个内存区域中是否包含另一个内存区域,函数原型如下:
```cpp
void *memmem(const void *haystack, size_t haystacklen, const void *needle, size_t needlelen);
```
其中,`haystack` 参数是要查找的内存区域,`haystacklen` 参数是 haystack 的长度,`needle` 参数是要查找的另一个内存区域,`needlelen` 参数是 needle 的长度。函数返回值是指向第一个匹配到的内存区域的指针,如果没有匹配到则返回 `NULL`。
在上面的代码中,如果找到了 Comm_Head 和 Comm_End,则计算出它们在 buf 数组中的位置,并进行后续处理,比如将数据通过串口发送出去。注意,这里使用了指针来计算 Comm_Head 和 Comm_End 的位置,因为使用数组下标访问 buf 数组时,如果遇到 0x00 字符则会被认为是字符串的结束符,因此不能正确计算位置。
strstr 字符串数组中包含0x00
`strstr` 函数在遇到字符串数组中的 `0x00` 时会停止搜索,因为 `0x00` 是字符串的结束符。如果字符串数组中包含 `0x00`,则不能使用 `strstr` 函数进行搜索。
一种解决方法是使用 `memmem` 函数,该函数用于在内存块中搜索指定的子内存块。函数原型如下:
```c
void *memmem(const void *haystack, size_t haystacklen, const void *needle, size_t needlelen);
```
其中,`haystack` 为要搜索的内存块,`haystacklen` 为要搜索的内存块的长度,`needle` 为要查找的子内存块,`needlelen` 为要查找的子内存块的长度。函数返回值为指向第一次出现子内存块的指针,如果未找到则返回 `NULL`。
示例代码如下:
```c
byte data[6] = {0x12, 0x34, 0x00, 0x56, 0x78, 0x00}; // 待搜索的字符串数组
byte pattern[3] = {0x00, 0x56, 0x78}; // 要查找的子字符串数组
byte *ptr = (byte *)memmem(data, sizeof(data), pattern, sizeof(pattern)); // 在字符串数组中搜索子字符串数组
if (ptr != NULL) {
int position = ptr - data; // 计算子字符串在字符串数组中的位置
Serial.println(position);
} else {
Serial.println("not found");
}
```
在上面的示例代码中,`memmem` 函数的第一个参数 `data` 是要搜索的字符串数组,第二个参数 `sizeof(data)` 是要搜索的字符串数组的长度,第三个参数 `pattern` 是要查找的子字符串数组,第四个参数 `sizeof(pattern)` 是要查找的子字符串数组的长度。使用上面的代码示例,串口输出的内容为 `2`,表示子字符串在字符串数组中的位置为 2。
注意:`memmem` 函数在某些编译器中可能不可用,需要包含相应的头文件才能使用。例如,在 Arduino 中可以包含 `string.h` 头文件来使用 `memmem` 函数。
阅读全文
相关推荐
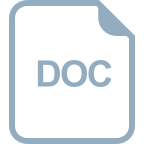


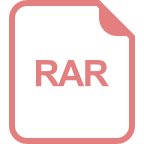
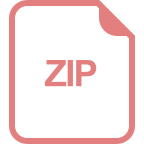
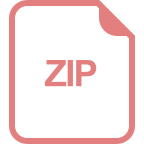
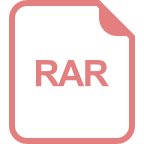
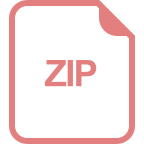
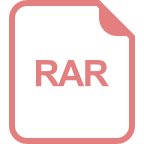
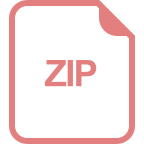