python脚本通过登重启录ambari页面开启关闭各服务,服务名称和操作可传入
时间: 2023-12-24 11:04:26 浏览: 29
可以使用 Ambari API 来实现 Python 脚本控制 Ambari 服务的启动和停止。以下是一个示例代码:
```python
import requests
import json
# Ambari 相关信息
ambari_host = 'your_ambari_host'
ambari_port = '8080'
ambari_user = 'your_ambari_username'
ambari_password = 'your_ambari_password'
# Ambari API 基础地址
ambari_api_base_url = 'http://{}:{}/api/v1'.format(ambari_host, ambari_port)
# 获取 Ambari 服务列表
def get_services():
url = '{}/services'.format(ambari_api_base_url)
response = requests.get(url, auth=(ambari_user, ambari_password))
services = json.loads(response.text)['items']
return services
# 获取指定服务的状态
def get_service_state(service_name):
url = '{}/services/{}/components'.format(ambari_api_base_url, service_name)
response = requests.get(url, auth=(ambari_user, ambari_password))
components = json.loads(response.text)['items']
for component in components:
if component['ServiceComponentInfo']['component_name'] == service_name + '_MASTER':
state = component['ServiceComponentInfo']['state']
return state
return None
# 启动指定服务
def start_service(service_name):
url = '{}/services/{}/'.format(ambari_api_base_url, service_name)
data = {"RequestInfo": {"context": "Start {} via Python".format(service_name)}, "Body": {"ServiceInfo": {"state": "STARTED"}}}
response = requests.put(url, auth=(ambari_user, ambari_password), json=data)
return response.status_code
# 停止指定服务
def stop_service(service_name):
url = '{}/services/{}/'.format(ambari_api_base_url, service_name)
data = {"RequestInfo": {"context": "Stop {} via Python".format(service_name)}, "Body": {"ServiceInfo": {"state": "INSTALLED"}}}
response = requests.put(url, auth=(ambari_user, ambari_password), json=data)
return response.status_code
# 示例:启动 HDFS 服务
services = get_services()
for service in services:
if service['ServiceInfo']['service_name'] == 'HDFS':
if get_service_state('HDFS') == 'STARTED':
print('HDFS service is already started.')
else:
print('Starting HDFS service...')
start_service('HDFS')
break
```
以上代码通过 Ambari API 获取服务列表和服务状态,并提供了启动和停止服务的方法。你可以根据需要传入不同的服务名称来控制服务的启动和停止。
相关推荐
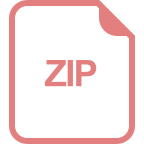
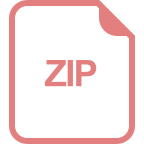














