java通过登录ambari页面重启各服务。服务名称可传入
时间: 2023-09-09 08:14:28 浏览: 109
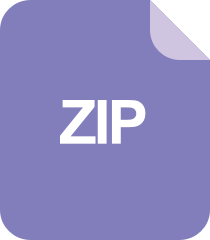
基于Python和Shell的Ambari自定义服务集成设计源码
您可以使用 Ambari 的 REST API 通过 Java 代码来重启服务。以下是一个示例代码片段:
```java
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpDelete;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.json.JSONObject;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class AmbariServiceRestart {
private static final String AMBARI_SERVER_URL = "http://your.ambari.server:8080";
private static final String CLUSTER_NAME = "your-cluster-name";
private static final String USERNAME = "your-username";
private static final String PASSWORD = "your-password";
public static void main(String[] args) {
CloseableHttpClient httpClient = HttpClients.createDefault();
// 登录 Ambari
String ambariSessionId = login(httpClient, AMBARI_SERVER_URL, USERNAME, PASSWORD);
// 获取集群服务
String services = getServices(httpClient, AMBARI_SERVER_URL, CLUSTER_NAME, ambariSessionId);
// 重启指定服务
restartService(httpClient, AMBARI_SERVER_URL, CLUSTER_NAME, ambariSessionId, "HDFS");
// 退出 Ambari
logout(httpClient, AMBARI_SERVER_URL, ambariSessionId);
try {
httpClient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private static String login(CloseableHttpClient httpClient, String ambariServerUrl, String username,
String password) {
String sessionId = null;
String loginUrl = ambariServerUrl + "/api/v1/session";
HttpPost httpPost = new HttpPost(loginUrl);
httpPost.setHeader("X-Requested-By", "ambari");
httpPost.setHeader("Content-Type", "application/json");
httpPost.setHeader("Accept", "application/json");
JSONObject requestBody = new JSONObject();
requestBody.put("username", username);
requestBody.put("password", password);
StringEntity requestEntity = new StringEntity(requestBody.toString(), "UTF-8");
httpPost.setEntity(requestEntity);
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
BufferedReader reader = new BufferedReader(
new InputStreamReader(response.getEntity().getContent()));
String inputLine;
StringBuffer responseBuffer = new StringBuffer();
while ((inputLine = reader.readLine()) != null) {
responseBuffer.append(inputLine);
}
JSONObject jsonResponse = new JSONObject(responseBuffer.toString());
sessionId = jsonResponse.getString("session_id");
} catch (IOException e) {
e.printStackTrace();
}
return sessionId;
}
private static void logout(CloseableHttpClient httpClient, String ambariServerUrl, String sessionId) {
String logoutUrl = ambariServerUrl + "/api/v1/logout";
HttpDelete httpDelete = new HttpDelete(logoutUrl);
httpDelete.setHeader("X-Requested-By", "ambari");
httpDelete.setHeader("Cookie", "AMBARISESSIONID=" + sessionId);
try {
httpClient.execute(httpDelete);
} catch (IOException e) {
e.printStackTrace();
}
}
private static String getServices(CloseableHttpClient httpClient, String ambariServerUrl, String clusterName,
String sessionId) {
String services = null;
String servicesUrl = ambariServerUrl + "/api/v1/clusters/" + clusterName + "/services";
HttpGet httpGet = new HttpGet(servicesUrl);
httpGet.setHeader("X-Requested-By", "ambari");
httpGet.setHeader("Cookie", "AMBARISESSIONID=" + sessionId);
try (CloseableHttpResponse response = httpClient.execute(httpGet)) {
BufferedReader reader = new BufferedReader(
new InputStreamReader(response.getEntity().getContent()));
String inputLine;
StringBuffer responseBuffer = new StringBuffer();
while ((inputLine = reader.readLine()) != null) {
responseBuffer.append(inputLine);
}
services = responseBuffer.toString();
} catch (IOException e) {
e.printStackTrace();
}
return services;
}
private static void restartService(CloseableHttpClient httpClient, String ambariServerUrl, String clusterName,
String sessionId, String serviceName) {
String restartUrl = ambariServerUrl + "/api/v1/clusters/" + clusterName + "/services/" + serviceName;
HttpPost httpPost = new HttpPost(restartUrl);
httpPost.setHeader("X-Requested-By", "ambari");
httpPost.setHeader("Content-Type", "application/json");
httpPost.setHeader("Accept", "application/json");
httpPost.setHeader("Cookie", "AMBARISESSIONID=" + sessionId);
JSONObject requestBody = new JSONObject();
requestBody.put("RequestInfo", new JSONObject().put("command", "RESTART"));
requestBody.put("ServiceInfo", new JSONObject().put("state", "INSTALLED"));
StringEntity requestEntity = new StringEntity(requestBody.toString(), "UTF-8");
httpPost.setEntity(requestEntity);
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
BufferedReader reader = new BufferedReader(
new InputStreamReader(response.getEntity().getContent()));
String inputLine;
StringBuffer responseBuffer = new StringBuffer();
while ((inputLine = reader.readLine()) != null) {
responseBuffer.append(inputLine);
}
System.out.println(responseBuffer.toString());
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
此示例代码通过 `login()` 方法登录 Ambari,并获取当前集群的服务列表;然后使用 `restartService()` 方法重启指定服务;最后使用 `logout()` 方法退出 Ambari。
请注意,您需要将 `AMBARI_SERVER_URL`、`CLUSTER_NAME`、`USERNAME` 和 `PASSWORD` 替换为您的 Ambari 服务器 URL、集群名称、用户名和密码。另外,如果您要重启多个服务,只需多次调用 `restartService()` 方法即可。
阅读全文
相关推荐
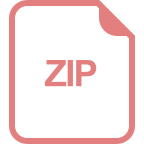
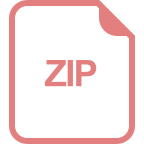







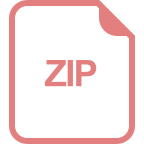







