MongoDB的模糊查询sql
时间: 2023-07-14 13:04:00 浏览: 243
MongoDB是一种文档型数据库,不使用SQL语言进行查询,而是使用自己的查询语言MongoDB Query Language (MQL)。在MongoDB中进行模糊查询可以使用正则表达式来实现。
以下是一个示例,演示如何在MongoDB中执行模糊查询:
```
db.collection.find({ fieldname: { $regex: /pattern/ } })
```
其中,`collection`是你的集合名称,`fieldname`是你要进行模糊查询的字段名,`pattern`是你要匹配的模式。
例如,假设我们有一个集合名为`users`,其中有一个字段`name`,我们想要查找名字中包含"John"的用户,可以使用以下查询语句:
```
db.users.find({ name: { $regex: /John/ } })
```
这将返回名字中包含"John"的所有用户文档。
需要注意的是,MongoDB的模糊查询对性能可能会有一定影响,因此在设计数据模型时需要谨慎考虑。另外,如果需要更高级的模糊查询功能,可以使用MongoDB提供的全文搜索引擎MongoDB Atlas Full-Text Search或第三方插件如Elasticsearch等来实现。
相关问题
mongodb模糊查询sql
MongoDB是一种非关系型数据库,它使用BSON(二进制JSON)格式存储数据。在MongoDB中,模糊查询可以通过正则表达式来实现。
在MongoDB中,可以使用$regex操作符进行模糊查询。$regex操作符接受一个正则表达式作为参数,用于匹配字段的值。以下是一个示例:
```
db.collection.find({ field: { $regex: /pattern/ } })
```
其中,`collection`是集合的名称,`field`是要匹配的字段名,`pattern`是正则表达式模式。
例如,如果要查询名字中包含"John"的所有文档,可以使用以下查询语句:
```
db.users.find({ name: { $regex: /John/ } })
```
这将返回所有名字中包含"John"的用户文档。
java mongodb 模糊查询 右like
在 MongoDB 中,没有像 SQL 中的 "LIKE" 操作符一样直接实现模糊查询。但是,我们可以使用正则表达式来实现类似的功能。
在 Java 中使用 MongoDB 进行右侧模糊查询,我们可以通过构建一个正则表达式来实现。下面是一个示例代码:
```java
import com.mongodb.client.FindIterable;
import com.mongodb.client.MongoCollection;
import com.mongodb.client.MongoDatabase;
import com.mongodb.client.model.Filters;
import org.bson.Document;
import com.mongodb.MongoClient;
import com.mongodb.MongoCredential;
public class Main {
public static void main(String[] args) {
// 连接到 MongoDB
MongoClient mongoClient = new MongoClient("localhost", 27017);
// 认证(如果需要)
MongoCredential credential = MongoCredential.createCredential("username", "database", "password".toCharArray());
MongoDatabase database = mongoClient.getDatabase("database");
// 获取集合
MongoCollection<Document> collection = database.getCollection("collectionName");
// 构建正则表达式
String keyword = "example";
String regexPattern = String.format(".*%s$", keyword);
// 构建查询条件
Document query = new Document("fieldName", new Document("$regex", regexPattern));
// 执行查询
FindIterable<Document> result = collection.find(query);
// 遍历结果
for (Document document : result) {
// 处理查询结果
System.out.println(document.toJson());
}
// 关闭连接
mongoClient.close();
}
}
```
在上述代码中,我们首先连接到 MongoDB,然后获取指定的集合。接下来,我们通过构建正则表达式 `.*example$`,其中 `example` 是我们想要匹配的关键字,并且右侧匹配。然后,我们使用 `$regex` 操作符将正则表达式作为查询条件传递给 `collection.find()` 方法,执行查询并返回结果。最后,我们可以对查询结果进行处理或输出。
需要注意的是,使用正则表达式进行模糊查询可能会对查询性能产生一定的影响,特别是对于大型数据集。因此,建议在需要模糊查询时谨慎使用,并根据实际情况进行性能测试和优化。
阅读全文
相关推荐
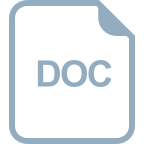
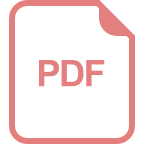
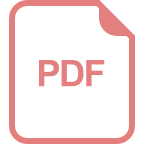
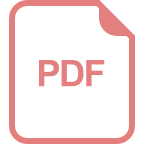
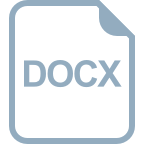
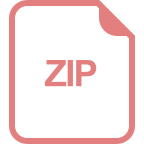
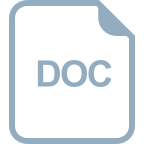
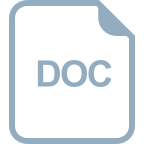
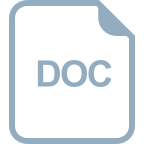
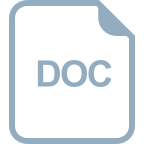
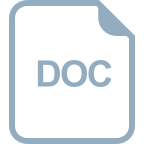
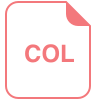
