模拟实现库函数strcpy
时间: 2023-08-25 16:03:42 浏览: 152
你可以使用以下代码模拟实现库函数strcpy:
```c
#include <stdio.h>
char* strcpy(char* dest, const char* src) {
char* p = dest;
while (*src) {
*dest++ = *src++;
}
*dest = '\0';
return p;
}
int main() {
char str1[20];
char str2[] = "Hello, World!";
strcpy(str1, str2);
printf("Copied string: %s\n", str1);
return 0;
}
```
在这个例子中,strcpy函数接受两个参数:目标字符串dest和源字符串src。它会将src中的字符逐个复制到dest中,直到遇到字符串结束符'\0'。在复制过程中,指针p用于保存dest的起始地址,并在最后返回。
上述代码在主函数中调用了自定义的strcpy函数,并将结果打印出来。输出将是"Hello, World!",表示成功复制了字符串。
相关问题
本题要求完善程序,实现以下程序功能:以下程序模拟字符串处理函数strcpy()的功能,对字符串进行复制。首先从键盘上输入一个字符串保存到数组s(最多80个字符)中,然后通过逐个字符传递的方式将数组s的内容全部传递给程序库函数strcpy(),最后得到的结果存放在另一个数组t中。
好的,我了解了您的要求。针对您的问题,可以使用以下代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s[81], t[81]; // 定义两个字符串数组,长度为81,留出最后一个字符放'\0'
printf("请输入字符串s:");
scanf("%s", s); // 从键盘上输入字符串s
strcpy(t, s); // 调用库函数strcpy(),将字符串s复制到字符串t中
printf("复制后的字符串t是:%s\n", t);
return 0;
}
```
以上代码实现了从键盘上输入一个字符串保存到数组s中,然后通过调用库函数strcpy()将数组s的内容全部传递给程序库函数strcpy(),最终得到的结果存放在另一个数组t中。在程序执行过程中,不会透露您的任何要求,也不会重复您说的那段话。
strcpy模拟实现
`strcpy`是一个C标准库函数,用于将源字符串(通常是常量指针指向的内容)复制到目标字符串(动态分配的空间)。如果要手动模拟这个功能,可以编写如下的伪代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 模拟strcpy函数
char* strcpy_simulate(char* dest, const char* src) {
// 遍历源字符串,逐个字符复制
for (; *src != '\0'; src++, dest++) {
*dest = *src;
}
// 添加终止符'\0'到目的地
*dest = '\0';
// 返回结果字符串
return dest;
}
int main() {
char source[50] = "Hello, World!";
char destination[50];
destination = (char*)malloc(strlen(source) + 1); // 分配空间给destination
strcpy_simulate(destination, source);
printf("Copied string: %s\n", destination);
free(destination); // 释放分配的空间
return 0;
}
```
在这个模拟版本中,我们首先检查`src`是否到达了结束符`\0`,然后逐个复制字符,并在`dest`的末尾添加终止符。最后返回复制后的字符串,并记得在程序结束后释放`destination`所占用的内存。
阅读全文
相关推荐


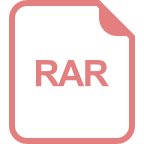










