模拟string类的封装
时间: 2023-12-08 10:04:23 浏览: 41
好的,以下是一个简单的模拟string类的封装示例:
```cpp
#include <iostream>
#include <cstring>
class myString {
private:
char* str;
int len;
public:
myString(const char* s = "") {
len = std::strlen(s);
str = new char[len + 1];
std::strcpy(str, s);
}
myString(const myString& other) {
len = other.len;
str = new char[len + 1];
std::strcpy(str, other.str);
}
~myString() {
delete[] str;
}
myString& operator=(const myString& other) {
if (this != &other) {
delete[] str;
len = other.len;
str = new char[len + 1];
std::strcpy(str, other.str);
}
return *this;
}
myString operator+(const myString& other) const {
char* temp = new char[len + other.len + 1];
std::strcpy(temp, str);
std::strcat(temp, other.str);
myString result(temp);
delete[] temp;
return result;
}
bool operator==(const myString& other) const {
return std::strcmp(str, other.str) == 0;
}
friend std::ostream& operator<<(std::ostream& os, const myString& s) {
os << s.str;
return os;
}
friend std::istream& operator>>(std::istream& is, myString& s) {
char temp[1024];
is >> temp;
s = myString(temp);
return is;
}
};
int main() {
myString s1("hello");
myString s2("world");
myString s3 = s1 + s2;
std::cout << s3 << std::endl;
std::cout << (s1 == s2) << std::endl;
std::cout << (s1 == myString("hello")) << std::endl;
std::cin >> s1;
std::cout << s1 << std::endl;
return 0;
}
```
在这个示例中,我们使用了一个char指针来存储字符串的内容,并使用了一个整数来存储字符串的长度。在构造函数中,我们使用了标准库函数strlen和strcpy来初始化字符串。在析构函数中,我们释放了分配的内存。在赋值运算符中,我们首先检查是否是自我赋值,然后释放当前分配的内存并复制新的字符串。在加法运算符中,我们创建了一个新的字符数组来存储两个字符串的连接,然后使用构造函数创建一个新的字符串对象并返回。在相等运算符中,我们使用标准库函数strcmp来比较两个字符串是否相等。最后,在流运算符中,我们使用标准库函数cin和cout来读取和输出字符串。
相关推荐
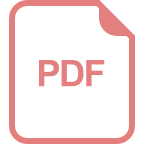
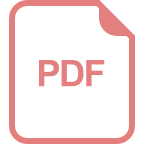
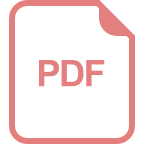














