利用Java实现一个程序,能够从屏幕接收输入,作为长方形的边和圆的半径,求长方形的边和圆形的面积
时间: 2024-10-14 11:17:40 浏览: 13
在Java中,我们可以使用`java.awt.event.KeyListener`接口来实现从键盘接收用户输入,并结合`Scanner`类来获取屏幕外的用户输入。这里是一个简单的示例程序,它创建了一个窗口,并监听用户的键盘输入,用于计算长方形和圆形的面积:
```java
import java.awt.*;
import javax.swing.*;
import java.util.Scanner;
public class AreaCalculator extends JFrame {
private JTextField lengthField;
private JTextField radiusField;
private JButton calculateButton;
private Scanner scanner = new Scanner(System.in);
public AreaCalculator() {
setTitle("Area Calculator");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(2, 2));
JLabel labelLength = new JLabel("Enter rectangle's length:");
lengthField = new JTextField(10);
labelRadius = new JLabel("Enter circle's radius:");
radiusField = new JTextField(10);
calculateButton = new JButton("Calculate");
calculateButton.addActionListener(e -> {
double length = Double.parseDouble(lengthField.getText());
double radius = Double.parseDouble(radiusField.getText());
double rectArea = length * width; // 假设已知宽度为width
double circArea = Math.PI * radius * radius;
System.out.println("Rectangle area: " + rectArea);
System.out.println("Circle area: " + circArea);
});
panel.add(labelLength);
panel.add(lengthField);
panel.add(labelRadius);
panel.add(radiusField);
panel.add(calculateButton);
add(panel);
setVisible(true);
}
public static void main(String[] args) {
new AreaCalculator();
}
}
// 用户无法直接在窗口内输入数字,所以我们还需要在键盘上获取额外的输入
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_ENTER) { // 按回车键提交输入
String input = scanner.nextLine(); // 获取当前输入行
if (input.contains("length")) {
lengthField.setText(input.split(" ")[1]); // 设置长度字段
} else if (input.contains("radius")) {
radiusField.setText(input.split(" ")[1]); // 设置半径字段
}
}
}
阅读全文
相关推荐
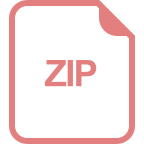
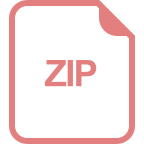
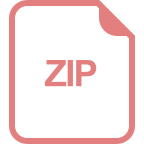



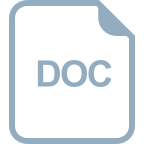
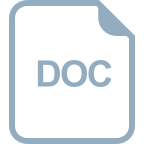
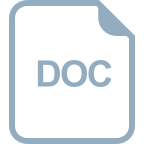




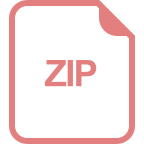
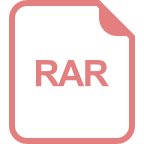