c语言写udp接收代码,需要统计时间跟数量
时间: 2024-01-18 09:05:02 浏览: 73
以下是一个简单的 C 语言程序,它使用 UDP 协议来接收数据报,同时统计接收到的数据报数量和接收所用的时间:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <sys/time.h>
#define MAX_BUF_SIZE 1024
int main(int argc, char *argv[]) {
if (argc != 2) {
fprintf(stderr, "Usage: %s port\n", argv[0]);
exit(1);
}
int sockfd = socket(AF_INET, SOCK_DGRAM, 0);
if (sockfd < 0) {
perror("socket");
exit(1);
}
struct sockaddr_in addr;
memset(&addr, 0, sizeof(addr));
addr.sin_family = AF_INET;
addr.sin_addr.s_addr = htonl(INADDR_ANY);
addr.sin_port = htons(atoi(argv[1]));
if (bind(sockfd, (struct sockaddr *)&addr, sizeof(addr)) < 0) {
perror("bind");
exit(1);
}
char buf[MAX_BUF_SIZE];
struct sockaddr_in client_addr;
socklen_t client_addrlen = sizeof(client_addr);
int count = 0;
struct timeval start_time, end_time;
gettimeofday(&start_time, NULL);
while (1) {
int len = recvfrom(sockfd, buf, MAX_BUF_SIZE, 0,
(struct sockaddr *)&client_addr, &client_addrlen);
if (len < 0) {
perror("recvfrom");
continue;
}
count++;
printf("Received data from %s:%d, size=%d\n",
inet_ntoa(client_addr.sin_addr), ntohs(client_addr.sin_port), len);
}
gettimeofday(&end_time, NULL);
close(sockfd);
double elapsed_time = (end_time.tv_sec - start_time.tv_sec) +
(end_time.tv_usec - start_time.tv_usec) / 1000000.0;
printf("Received %d packets in %.3f seconds\n", count, elapsed_time);
return 0;
}
```
该程序首先创建一个 UDP 套接字,然后将其绑定到指定的端口上。接着,它使用循环来接收来自客户端的数据报,并统计接收到的数据报数量。最后,它计算接收所用的时间,并打印出统计结果。
阅读全文
相关推荐




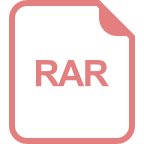
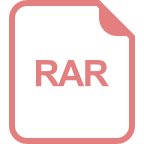







