汽车租赁系统V1.0版,每天租金500元,输入租赁天数,输出租赁总金额。java代码
时间: 2023-05-14 14:04:52 浏览: 129
以下是汽车租赁系统V1.0版的Java代码:
```
import java.util.Scanner;
public class CarRentalSystem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入租赁天数:");
int days = scanner.nextInt();
int total = days * 500;
System.out.println("租赁总金额为:" + total + "元");
}
}
```
您可以通过输入租赁天数来计算租赁总金额。每天的租金为500元。
相关问题
写一个汽车租赁系统的Java代码
好的,我可以为您提供一个简单的汽车租赁系统的Java代码,具体如下所示:
```
import java.util.Scanner;
public class CarRentalSystem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String carType;
int rentalPeriod;
int totalPrice;
System.out.println("欢迎使用汽车租赁系统!");
System.out.print("请输入您希望租赁的车辆类型:");
carType = scanner.nextLine();
System.out.print("请输入租赁时长(天数):");
rentalPeriod = scanner.nextInt();
if (carType.equals("经济型")) {
totalPrice = rentalPeriod * 100;
} else if (carType.equals("商务型")) {
totalPrice = rentalPeriod * 200;
} else if (carType.equals("豪华型")) {
totalPrice = rentalPeriod * 300;
} else {
System.out.println("对不起,您输入的车辆类型不存在!");
return;
}
System.out.println("您租赁的" + carType + "车辆,共需支付" + totalPrice + "元。");
}
}
```
在这个代码中,用户可以输入希望租赁的车辆类型和租赁时长,然后根据不同类型的车辆计算总价并输出。如果用户输入的车辆类型不存在,程序会显示错误信息并结束。
用Java写一个汽车租赁系统的代码,要用到GUI
这里给出一个简单的汽车租赁系统的Java代码实现,包含了基本的GUI界面。可以根据需求进行修改和扩展。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
public class CarRentalSystem extends JFrame implements ActionListener {
private ArrayList<Car> cars; // 汽车列表
private JComboBox<String> carComboBox; // 汽车下拉框
private JTextField rentDaysTextField; // 租车天数文本框
private JLabel priceLabel; // 租车价格标签
public CarRentalSystem() {
cars = new ArrayList<>();
cars.add(new Car("Toyota", "Camry", 300));
cars.add(new Car("Honda", "Accord", 350));
cars.add(new Car("Ford", "Mustang", 400));
// 创建界面
JPanel mainPanel = new JPanel(new GridLayout(4, 2));
JLabel carLabel = new JLabel("选择汽车");
carComboBox = new JComboBox<>();
for (Car car : cars) {
carComboBox.addItem(car.toString());
}
mainPanel.add(carLabel);
mainPanel.add(carComboBox);
JLabel rentDaysLabel = new JLabel("租车天数");
rentDaysTextField = new JTextField();
mainPanel.add(rentDaysLabel);
mainPanel.add(rentDaysTextField);
JButton rentButton = new JButton("租车");
rentButton.addActionListener(this);
mainPanel.add(rentButton);
JLabel priceTextLabel = new JLabel("租金");
priceLabel = new JLabel();
mainPanel.add(priceTextLabel);
mainPanel.add(priceLabel);
add(mainPanel, BorderLayout.CENTER);
setTitle("汽车租赁系统");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
new CarRentalSystem();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("租车")) {
int index = carComboBox.getSelectedIndex();
Car car = cars.get(index);
int days = Integer.parseInt(rentDaysTextField.getText());
int price = car.rent(days);
priceLabel.setText(String.valueOf(price));
}
}
}
class Car {
private String make; // 品牌
private String model; // 型号
private int dailyPrice; // 每日租金
public Car(String make, String model, int dailyPrice) {
this.make = make;
this.model = model;
this.dailyPrice = dailyPrice;
}
public int rent(int days) {
return days * dailyPrice;
}
@Override
public String toString() {
return make + " " + model;
}
}
```
这个代码实现了一个简单的汽车租赁系统,用户可以选择要租赁的汽车和租车天数,系统会根据选择计算出租金并显示在界面上。在这个示例中,使用了JComboBox、JTextField、JButton和JLabel等Swing组件来构建GUI界面。可以根据需求进行修改和扩展。
相关推荐
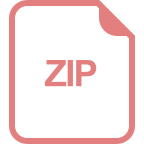












