用Java写一个日历加钟表要带图形界面
时间: 2024-05-15 21:12:36 浏览: 12
好的,我可以给你提供一个简单的Java代码示例,使用Swing库来实现一个日历和时钟的图形界面。
```java
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.Timer;
public class CalendarClock extends JFrame {
private static final long serialVersionUID = 1L;
private JPanel calendarPanel;
private JPanel clockPanel;
private JLabel dateLabel;
private JLabel timeLabel;
private SimpleDateFormat dateFormat;
private SimpleDateFormat timeFormat;
public CalendarClock() {
super("Calendar and Clock");
// Set up date and time formats
dateFormat = new SimpleDateFormat("EEE, MMM d, yyyy");
timeFormat = new SimpleDateFormat("h:mm:ss a");
// Create calendar panel
calendarPanel = new JPanel(new GridLayout(7, 7));
calendarPanel.setBackground(Color.WHITE);
// Add day labels
String[] daysOfWeek = {"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"};
for (int i = 0; i < daysOfWeek.length; i++) {
JLabel label = new JLabel(daysOfWeek[i], JLabel.CENTER);
label.setFont(new Font("SansSerif", Font.BOLD, 14));
calendarPanel.add(label);
}
// Add calendar days
Calendar cal = Calendar.getInstance();
cal.set(Calendar.DAY_OF_MONTH, 1);
int firstDayOfWeek = cal.get(Calendar.DAY_OF_WEEK);
int daysInMonth = cal.getActualMaximum(Calendar.DAY_OF_MONTH);
int dayOfMonth = 1 - firstDayOfWeek + 1;
for (int i = 0; i < 7 * 6; i++) {
if (i < firstDayOfWeek - 1 || dayOfMonth > daysInMonth) {
calendarPanel.add(new JLabel(""));
} else {
JLabel label = new JLabel("" + dayOfMonth, JLabel.CENTER);
label.setFont(new Font("SansSerif", Font.PLAIN, 14));
calendarPanel.add(label);
dayOfMonth++;
}
}
// Create clock panel
clockPanel = new JPanel(new BorderLayout());
clockPanel.setBackground(Color.WHITE);
// Add date label
dateLabel = new JLabel(dateFormat.format(new Date()), JLabel.CENTER);
dateLabel.setFont(new Font("SansSerif", Font.BOLD, 18));
clockPanel.add(dateLabel, BorderLayout.NORTH);
// Add time label
timeLabel = new JLabel(timeFormat.format(new Date()), JLabel.CENTER);
timeLabel.setFont(new Font("SansSerif", Font.BOLD, 24));
clockPanel.add(timeLabel, BorderLayout.CENTER);
// Create timer to update time label
Timer timer = new Timer(1000, new ActionListener() {
public void actionPerformed(ActionEvent e) {
timeLabel.setText(timeFormat.format(new Date()));
}
});
timer.start();
// Add panels to frame
add(calendarPanel, BorderLayout.WEST);
add(clockPanel, BorderLayout.CENTER);
// Set frame size and visibility
setSize(600, 400);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
new CalendarClock();
}
}
```
这个程序会在左侧显示一个日历,右侧显示一个时钟,并且会每秒更新一次当前时间。你可以根据自己的需求对代码进行修改和定制。
相关推荐
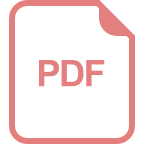
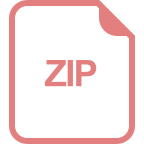













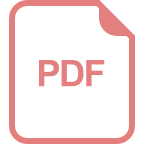