单链表在C++中如何使用?能写段代码举例吗?
时间: 2023-03-14 10:33:18 浏览: 132
在C中使用单链表可以通过定义结构体和定义指针,来表示每个节点,然后通过指针变量将节点串联起来。下面是一段示例代码:struct node{
int data;
struct node* next;
};
// Utility function to create a new node
struct node* newNode(int data)
{
struct node* node = (struct node*)malloc(sizeof(struct node));
node->data = data;
node->next = NULL;
return node;
}
相关问题
有头单链表怎么变成无头单链 表 详细举例 不适用C++
假设有一个头节点为 head,我们需要将它变成无头单链表。
1. 首先,我们定义一个指针变量 p,让它指向头节点。
2. 然后,我们将头节点的指针域赋值给头节点,即 head = head->next。
3. 最后,我们释放指针变量 p 的内存空间,即 free(p)。
以下是详细的代码实现:
```c
// 定义单链表结构体
typedef struct Node {
int data;
struct Node *next;
} Node;
// 将头节点变为普通节点
void removeHeadNode(Node **head) {
if (*head == NULL) {
return;
}
Node *p = *head;
*head = (*head)->next;
free(p);
}
```
在上面的代码中,我们首先定义了一个单链表结构体 Node,其中包含了数据域 data 和指针域 next。
然后,我们定义了一个函数 removeHeadNode,这个函数接受一个指向头节点指针的指针,即指向指针的指针。这样做是因为我们需要修改头节点指针的值,而指针是传值调用的,不会对原指针进行修改。
在函数中,我们首先对头指针进行非空判断,然后定义一个指针变量 p 并让它指向头节点。接着,我们将头节点的指针域赋值给头节点,即 head = head->next,这样就把头节点变成了普通节点。最后,我们释放指针变量 p 的内存空间,即 free(p)。
这样,我们就将一个带头节点的单链表变成了一个不带头节点的单链表。
有头单链表怎么变成无头单链 表 详细举例
将一个有头单链表变成无头单链表,需要做以下几个步骤:
1. 定义一个新的指针变量,指向原有头结点的下一个节点,即第一个有效节点。
2. 释放原有头结点的内存空间,使新的指针变量成为链表的新头结点。
3. 修改链表中所有节点的指针域,使它们都指向下一个节点而不是当前节点的前一个节点。
下面是一个具体的示例:
假设有一个有头单链表L,其中头结点的数据域为-1,指针域指向第一个有效节点1,而第一个有效节点1的数据域为10,指针域指向第二个有效节点2。链表中共有5个节点,最后一个节点的指针域为NULL。
```
头结点 第一个有效节点 第二个有效节点 第三个有效节点 第四个有效节点 第五个有效节点
-1 -> 1(10) -> 2(20) -> 3(30) -> 4(40) -> 5(50) -> NULL
```
现在要将这个有头单链表变成无头单链表,具体的步骤如下:
1. 定义一个新的指针变量p,指向第一个有效节点1。
2. 释放原有头结点的内存空间。
3. 修改每个节点的指针域,使它们都指向下一个节点。
代码实现如下:
```c++
// 定义有头单链表的结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 将有头单链表变成无头单链表
ListNode* removeHead(ListNode* head) {
if (head == NULL || head->next == NULL) {
return head;
}
ListNode* p = head->next; // 新的头结点指向第一个有效节点
delete head; // 释放原有头结点的内存空间
head = p; // 新的头结点是第一个有效节点
while (p != NULL) { // 修改每个节点的指针域
ListNode* next = p->next;
p->next = head;
head = p;
p = next;
}
return head;
}
```
执行上述代码后,链表就变成了无头单链表,其结构如下:
```
第一个有效节点 第二个有效节点 第三个有效节点 第四个有效节点 第五个有效节点
1(10) -> 2(20) -> 3(30) -> 4(40) -> 5(50) -> NULL
```
阅读全文
相关推荐




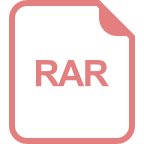





