用c++写单链表代码
时间: 2024-01-27 20:03:37 浏览: 74
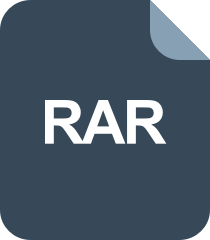
单链表的C++代码
以下是C++实现单链表的示例代码:
```c++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
// 定义链表类
class LinkedList {
private:
ListNode* head;
public:
LinkedList() {
head = NULL;
}
// 在链表末尾添加节点
void append(int val) {
ListNode* node = new ListNode(val);
if (head == NULL) {
head = node;
} else {
ListNode* curr = head;
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = node;
}
}
// 在链表指定位置插入节点
void insert(int val, int pos) {
ListNode* node = new ListNode(val);
if (pos == 0) {
node->next = head;
head = node;
} else {
ListNode* curr = head;
for (int i = 0; i < pos - 1; i++) {
if (curr == NULL) {
cout << "插入位置超出链表长度" << endl;
return;
}
curr = curr->next;
}
node->next = curr->next;
curr->next = node;
}
}
// 删除链表指定位置的节点
void remove(int pos) {
if (pos == 0) {
head = head->next;
} else {
ListNode* curr = head;
for (int i = 0; i < pos - 1; i++) {
if (curr == NULL || curr->next == NULL) {
cout << "删除位置超出链表长度" << endl;
return;
}
curr = curr->next;
}
curr->next = curr->next->next;
}
}
// 获取链表指定位置的节点值
int get(int pos) {
ListNode* curr = head;
for (int i = 0; i < pos; i++) {
if (curr == NULL) {
cout << "获取位置超出链表长度" << endl;
return -1;
}
curr = curr->next;
}
return curr->val;
}
// 获取链表长度
int size() {
int count = 0;
ListNode* curr = head;
while (curr != NULL) {
count++;
curr = curr->next;
}
return count;
}
// 打印链表
void print() {
ListNode* curr = head;
while (curr != NULL) {
cout << curr->val << " ";
curr = curr->next;
}
cout << endl;
}
};
int main() {
LinkedList list;
list.append(1);
list.append(2);
list.append(3);
list.print(); // 输出: 1 2 3
list.insert(4, 1);
list.print(); // 输出: 1 4 2 3
list.remove(2);
list.print(); // 输出: 1 4 3
cout << list.get(1) << endl; // 输出: 4
cout << list.size() << endl; // 输出: 3
return 0;
}
```
在上述代码中,我们定义了链表节点结构体`ListNode`,其中包含一个整型变量`val`和一个指向下一个节点的指针`next`;定义了链表类`LinkedList`,其中包含各种操作链表的函数,例如:在链表末尾添加节点、在链表指定位置插入节点、删除链表指定位置的节点等等。在`main()`函数中,我们初始化了一个链表对象`list`,并对其进行了各种操作,最后输出了链表中的元素。
阅读全文
相关推荐
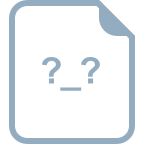

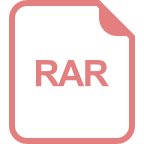

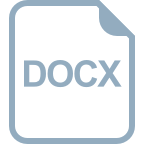
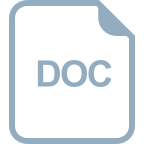









