用c++实现单链表的操作
时间: 2023-10-14 21:04:52 浏览: 78
以下是用C++实现单链表操作的示例代码:
```cpp
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct ListNode {
int val; // 存储节点的值
ListNode *next; // 指向下一个节点的指针
ListNode(int x) : val(x), next(NULL) {} // 构造函数
};
// 定义链表类
class LinkedList {
public:
LinkedList() { // 构造函数
head = NULL;
}
~LinkedList() { // 析构函数
ListNode *cur = head;
while (cur != NULL) {
ListNode *temp = cur;
cur = cur->next;
delete temp;
}
}
void add(int val) { // 添加节点
if (head == NULL) {
head = new ListNode(val);
} else {
ListNode *cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = new ListNode(val);
}
}
void remove(int val) { // 移除节点
if (head == NULL) {
return;
}
if (head->val == val) {
ListNode *temp = head;
head = head->next;
delete temp;
} else {
ListNode *cur = head;
while (cur->next != NULL && cur->next->val != val) {
cur = cur->next;
}
if (cur->next != NULL) {
ListNode *temp = cur->next;
cur->next = cur->next->next;
delete temp;
}
}
}
void print() { // 打印链表
ListNode *cur = head;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
}
private:
ListNode *head; // 链表头指针
};
int main() {
LinkedList list;
list.add(1);
list.add(2);
list.add(3);
list.print(); // 输出:1 2 3
list.remove(2);
list.print(); // 输出:1 3
return 0;
}
```
该代码实现了单链表的添加、移除和打印操作。需要注意的是,析构函数需要手动释放链表中所有节点的内存,否则可能会出现内存泄漏的问题。
阅读全文
相关推荐
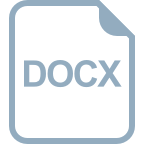
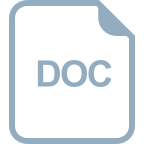
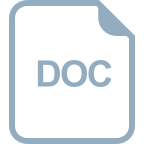
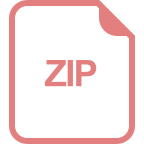
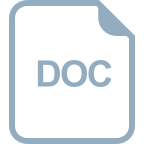
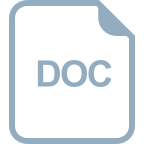
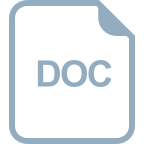
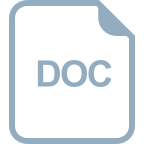
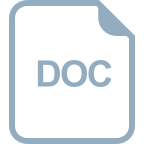

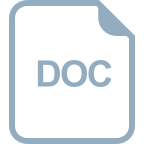
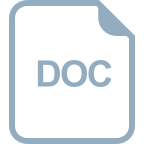
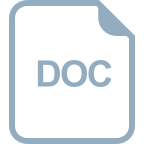
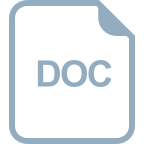
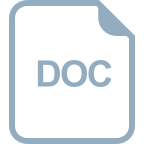
