单链表基本操作的实现C++
时间: 2023-10-19 07:07:00 浏览: 119
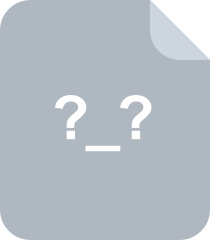
c++单链表基本操作的实现
单链表的基本操作包括插入、删除、查找和打印等。以下是C++实现单链表基本操作的代码示例:
```cpp
#include<iostream>
using namespace std;
// 定义单链表节点结构
struct ListNode {
int data;
ListNode* next;
};
// 头插法创建单链表
bool LinkedList_hCreate(ListNode*& head) {
head = new ListNode; // 创建头节点
head->next = NULL;
int num;
cout << "请输入节点数:";
cin >> num;
cout << "请输入节点数据:";
for (int i = 0; i < num; i++) {
ListNode* newNode = new ListNode;
cin >> newNode->data;
newNode->next = head->next;
head->next = newNode;
}
return true;
}
// 尾插法创建单链表
bool LinkedList_rCreate(ListNode*& head) {
head = new ListNode; // 创建头节点
head->next = NULL;
int num;
cout << "请输入节点数:";
cin >> num;
cout << "请输入节点数据:";
ListNode* rear = head;
for (int i = 0; i < num; i++) {
ListNode* newNode = new ListNode;
cin >> newNode->data;
rear->next = newNode;
rear = newNode;
}
rear->next = NULL;
return true;
}
// 打印单链表
void print(ListNode* head) {
ListNode* p = head->next;
while (p) {
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
int main() {
ListNode* head;
LinkedList_hCreate(head); // 调用头插法创建单链表函数
print(head); // 打印单链表
return 0;
}
```
阅读全文
相关推荐





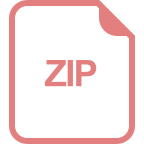
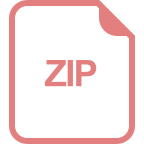
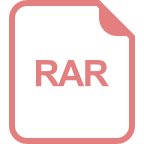
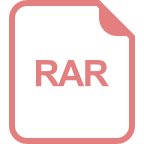
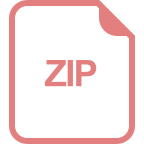
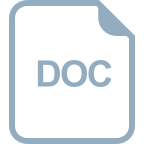
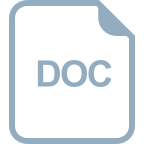
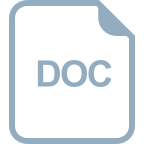

