单链表基本操作实现,包括初始化、创建单链表、取值、查找、插入、删除等操作。
时间: 2024-10-15 18:09:56 浏览: 41
单链表是一种线性数据结构,每个节点包含两个部分:存储数据的变量和指向下一个节点的指针。以下是单链表的基本操作实现:
1. **初始化**:
- 创建空链表:定义一个节点类,其中包含数据域和指针域,初始化链表头指针为`NULL`。
```c++
struct ListNode {
int data;
ListNode* next;
ListNode(int val = 0) : data(val), next(NULL) {}
};
```
2. **创建单链表**:
- 动态创建节点并链接:可以逐个添加元素到链表尾部。
```c++
ListNode* createList(int* arr, int n) {
ListNode* head = new ListNode(arr[0]);
for (int i = 1; i < n; i++) {
ListNode* newNode = new ListNode(arr[i]);
newNode->next = head;
head = newNode;
}
return head;
}
```
3. **取值**:
- 遍历链表,通过节点的`data`属性获取值。
```c++
int getValue(ListNode* node) {
if (node != NULL) {
return node->data;
} else {
return -1; // 或者抛出异常,表示无效访问
}
}
```
4. **查找**:
- 使用指针遍历链表,直到找到目标值或到达链表尾部。
```c++
ListNode* search(ListNode* head, int target) {
while (head && head->data != target) {
head = head->next;
}
return head;
}
```
5. **插入**:
- 在指定位置插入新节点。如果插入点是尾部,则类似创建链表;如果不是尾部,需要找到插入点并更新指针。
```c++
void insertNode(ListNode*& head, int value, int position) {
if (position == 0) {
ListNode* newNode = new ListNode(value);
newNode->next = head;
head = newNode;
} else {
ListNode* current = head;
for (int i = 0; i < position - 1 && current != NULL; ++i) {
current = current->next;
}
if (current != NULL) {
ListNode* newNode = new ListNode(value);
newNode->next = current->next;
current->next = newNode;
} else {
std::cout << "Invalid insertion position" << std::endl;
}
}
}
```
6. **删除**:
- 删除特定值的节点。这涉及两个步骤:找到待删除的节点,并更新前一个节点的`next`指针指向该节点的后一个节点。
```c++
void deleteNode(ListNode*& head, int target) {
if (head == NULL) {
return;
}
if (head->data == target) {
ListNode* temp = head;
head = head->next;
delete temp;
return;
}
ListNode* prev = head;
ListNode* curr = head->next;
while (curr != NULL && curr->data != target) {
prev = curr;
curr = curr->next;
}
if (curr != NULL) {
prev->next = curr->next;
delete curr;
} else {
std::cout << "Target not found in the list." << std::endl;
}
}
```
阅读全文
相关推荐
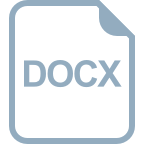
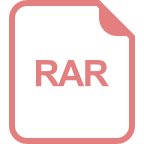
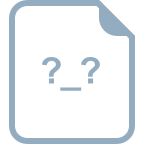















