单链表实现定义初始化插入删除查找的完整代码
时间: 2024-09-19 22:10:19 浏览: 49
在Python中,我们可以使用类来实现单链表的基本操作。这里是一个简单的例子,展示了如何创建单链表、初始化、插入节点、删除节点以及查找节点:
```python
class ListNode:
def __init__(self, data=0, next=None):
self.data = data
self.next = next
class LinkedList:
def __init__(self):
self.head = None
# 插入节点
def insert(self, data):
if not self.head:
self.head = ListNode(data)
else:
current = self.head
while current.next:
current = current.next
current.next = ListNode(data)
# 删除节点
def delete(self, key):
if not self.head:
return
if self.head.data == key:
self.head = self.head.next
return
current = self.head
while current.next and current.next.data != key:
current = current.next
if current.next:
current.next = current.next.next
# 查找节点
def find(self, key):
current = self.head
while current:
if current.data == key:
return True
current = current.next
return False
# 示例
linked_list = LinkedList()
linked_list.insert(1) # 初始化,插入节点1
linked_list.insert(2) # 插入节点2
print(linked_list.find(2)) # 查找节点2,返回True
# 删除节点2
linked_list.delete(2)
print(linked_list.find(2)) # 查找节点2,现在返回False
```
这个例子中,`ListNode`是链表的节点,而`LinkedList`包含了链表的操作方法如`insert`、`delete`和`find`。注意,这个实现假设了链表中元素是唯一的,如果存在相同的值,`delete`函数只删除第一次找到的节点。
阅读全文
相关推荐
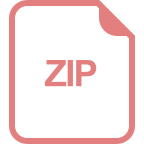
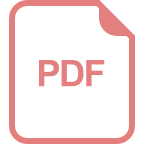
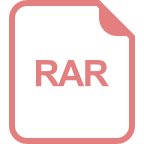

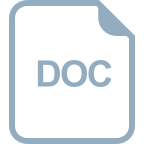
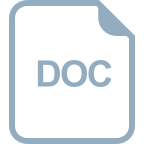
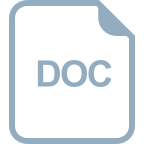


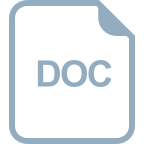
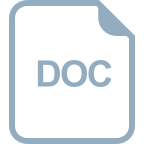
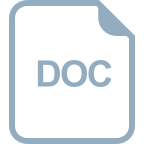






