单链表的初始化、插入、查找、删除完整代码
时间: 2024-09-27 12:08:57 浏览: 30
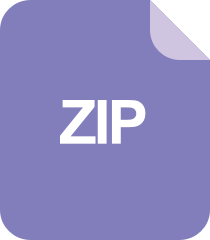
数据结构单链表初始化c/c++语言代码实现
以下是单链表的基本操作——初始化、插入、查找和删除的一个简化的示例,我们将用C语言来实现:
**初始化:**
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
struct Node {
int data;
struct Node* next;
};
// 初始化链表
struct Node* createList() {
struct Node* head = NULL;
return head;
}
int main() {
struct Node* list = createList();
if (list == NULL) {
printf("Failed to initialize the list.\n");
} else {
printf("List initialized successfully.\n");
}
return 0;
}
```
**插入:**
```c
void insert(struct Node** head, int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode) {
newNode->data = value;
newNode->next = *head;
*head = newNode;
} else {
printf("Memory allocation failed.\n");
}
}
// 在main中调用
insert(&list, 1);
```
**查找:**
```c
struct Node* search(struct Node* head, int target) {
struct Node* current = head;
while (current != NULL) {
if (current->data == target)
return current;
current = current->next;
}
return NULL;
}
int found = search(list, 1);
if (found)
printf("Found the element.\n");
else
printf("Element not found in the list.\n");
```
**删除:**
```c
void deleteNode(struct Node** head, int value) {
struct Node* current = *head, *previous = NULL;
if (current && current->data == value) {
*head = current->next;
free(current); // 释放当前节点
return;
}
while (current != NULL && current->data != value) {
previous = current;
current = current->next;
}
if (current == NULL) return; // 如果未找到要删除的节点
previous->next = current->next; // 更新前一个节点的指针
free(current); // 释放当前节点
}
// 在main中调用
deleteNode(&list, 1);
```
以上代码仅用于演示基本操作,实际项目中可能需要考虑更多边界条件和错误处理。
阅读全文
相关推荐
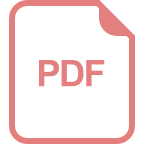

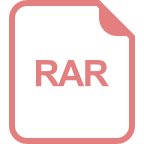
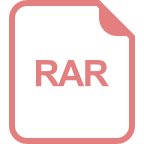
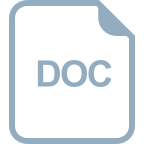
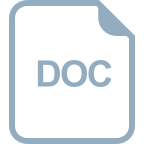
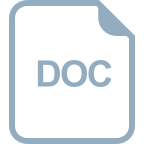









