循环单链表代码c++
时间: 2024-10-11 17:09:05 浏览: 36
循环单链表是一种特殊的链表结构,它的最后一个节点指向第一个节点,形成一个环形。在 C++ 中,你可以通过定义一个节点结构(Node)并创建头结点(head)来实现循环单链表。以下是基本的代码示例:
```cpp
#include <iostream>
// 定义链表节点结构体
struct Node {
int data;
Node* next; // 指向下一个节点的指针
};
class CircularLinkedList {
private:
Node* head; // 链表头节点
public:
// 构造函数
CircularLinkedList() : head(nullptr) {}
// 插入元素到链表头部
void insertAtBeginning(int value) {
if (head == nullptr) {
head = new Node{value, head};
} else {
Node* newNode = new Node{value, head->next};
head->next = newNode;
newNode->next = head;
}
}
// 打印链表内容(考虑循环特性)
void display() {
Node* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
if (temp == head) {
break; // 如果到达头节点,表示打印完一圈
}
}
std::cout << "\n";
}
};
int main() {
CircularLinkedList list;
list.insertAtBeginning(5);
list.insertAtBeginning(4);
list.insertAtBeginning(3);
list.display(); // 输出: 3 4 5
return 0;
}
```
阅读全文
相关推荐
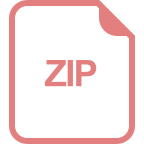
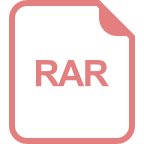
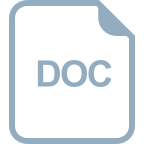
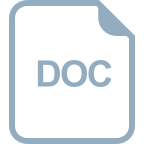
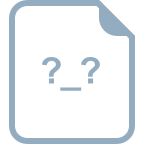
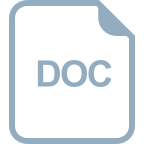
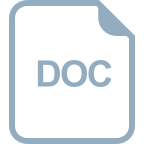









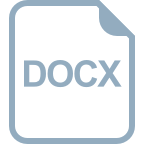