写一个关于循环单链表的实现与测试的C++代码
时间: 2024-09-28 21:06:55 浏览: 6
当然可以。首先,我们来创建一个简单的循环单链表节点结构,并提供插入、删除节点以及遍历的功能。接着,我们将编写一些测试函数来验证链表的正确性。
```cpp
#include <iostream>
// 定义循环单链表节点结构体
struct Node {
int data;
Node* next;
};
class CircularLinkedList {
private:
Node* head;
public:
// 构造函数,初始化空链表
CircularLinkedList() : head(nullptr) {}
// 插入节点到链表头部
void insert(int value) {
if (!head) {
head = new Node{value, head};
} else {
Node* newNode = new Node{value, nullptr};
newNode->next = head;
head->next = newNode;
head = newNode;
}
}
// 删除节点(仅支持从头开始删除)
void deleteNode(int value) {
if (head && head->data == value) {
Node* temp = head;
while (temp->next != head) {
temp = temp->next;
}
delete head;
head = nullptr;
} else {
Node* current = head;
while (current->next != head && current->next->data != value) {
current = current->next;
}
if (current->next->data == value) {
Node* toDelete = current->next;
current->next = toDelete->next;
delete toDelete;
}
}
}
// 遍历并打印链表
void display() const {
Node* temp = head;
if (head) {
do {
std::cout << temp->data << " -> ";
temp = temp->next;
} while (temp != head);
std::cout << "Head" << std::endl;
} else {
std::cout << "List is empty." << std::endl;
}
}
};
// 测试函数
void testCircularLinkedList(CircularLinkedList& list) {
// 插入元素
list.insert(1);
list.insert(2);
list.insert(3);
// 显示并确认元素
list.display();
// 删除元素
list.deleteNode(2);
// 再次显示确认元素已删除
list.display();
// 清空链表
while (list.head) {
list.deleteNode(list.head->data);
}
// 最后确认链表为空
list.display();
}
int main() {
CircularLinkedList CLL;
testCircularLinkedList(CLL);
return 0;
}
```
在这个例子中,`testCircularLinkedList` 函数用于创建一个循环链表,插入元素,删除元素并检查链表的状态。运行 `main` 函数可以看到整个过程。
相关推荐
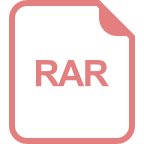
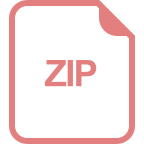
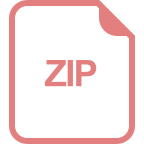














