帮我用js写一个贪吃蛇
时间: 2023-08-20 15:33:45 浏览: 46
好的,这里是一个简单的贪吃蛇的示例。它是使用HTML5 Canvas和JavaScript编写的。
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇</title>
<meta charset="utf-8">
<style type="text/css">
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="canvas" width="600" height="600"></canvas>
<script type="text/javascript" src="snake.js"></script>
</body>
</html>
```
JavaScript代码:
```javascript
// 定义画布和上下文
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
// 定义蛇的初始位置和方向
var snake = [{x: 10, y: 10}, {x: 9, y: 10}, {x: 8, y: 10}];
var direction = "right";
// 定义食物的初始位置
var food = {x: Math.floor(Math.random() * 30), y: Math.floor(Math.random() * 30)};
// 定义游戏循环
var gameLoop = setInterval(function() {
// 移动蛇的位置
var head = {x: snake[0].x, y: snake[0].y};
switch (direction) {
case "up":
head.y--;
break;
case "down":
head.y++;
break;
case "left":
head.x--;
break;
case "right":
head.x++;
break;
}
snake.unshift(head);
// 判断是否吃到食物
if (head.x === food.x && head.y === food.y) {
food = {x: Math.floor(Math.random() * 30), y: Math.floor(Math.random() * 30)};
} else {
snake.pop();
}
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 绘制食物
ctx.fillStyle = "red";
ctx.fillRect(food.x * 20, food.y * 20, 20, 20);
// 绘制蛇的身体
ctx.fillStyle = "green";
for (var i = 0; i < snake.length; i++) {
ctx.fillRect(snake[i].x * 20, snake[i].y * 20, 20, 20);
}
// 判断游戏是否结束
if (head.x < 0 || head.x >= 30 || head.y < 0 || head.y >= 30) {
clearInterval(gameLoop);
alert("游戏结束!");
}
for (var j = 1; j < snake.length; j++) {
if (head.x === snake[j].x && head.y === snake[j].y) {
clearInterval(gameLoop);
alert("游戏结束!");
}
}
}, 100);
// 监听键盘事件
document.addEventListener("keydown", function(event) {
switch (event.keyCode) {
case 37:
if (direction !== "right") {
direction = "left";
}
break;
case 38:
if (direction !== "down") {
direction = "up";
}
break;
case 39:
if (direction !== "left") {
direction = "right";
}
break;
case 40:
if (direction !== "up") {
direction = "down";
}
break;
}
});
```
这个示例中,我们使用了HTML5 Canvas来绘制游戏界面,使用了JavaScript来实现游戏逻辑。在游戏循环中,我们首先根据当前方向移动蛇的位置,然后判断是否吃到了食物,如果吃到了就重新生成一个新的食物,否则就删除蛇的最后一个元素。然后我们清空画布,绘制食物和蛇的身体。最后判断游戏是否结束,如果蛇的头部碰到边界或者碰到了自己的身体,就停止游戏并弹出游戏结束的提示框。同时,我们还监听了键盘事件,根据不同的按键来改变蛇的方向。
相关推荐
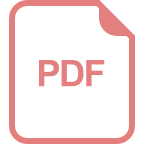








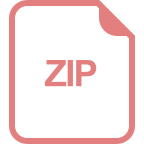
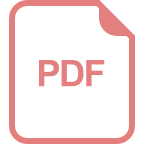
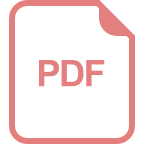
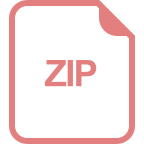