帮我用JS写一个贪吃蛇的游戏
时间: 2023-08-20 16:23:20 浏览: 46
好的,以下是一个基于HTML、CSS和JavaScript的简单贪吃蛇游戏的实现。
HTML代码:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>贪吃蛇游戏</title>
<style>
body {
font-family: sans-serif;
}
#game-container {
width: 600px;
height: 400px;
border: 1px solid #ddd;
margin: 0 auto;
position: relative;
}
#game-container .snake, #game-container .food {
position: absolute;
width: 20px;
height: 20px;
background-color: #333;
}
#game-container .snake {
border-radius: 10px;
}
#game-container .food {
border-radius: 50%;
}
#game-container .score {
position: absolute;
top: 10px;
right: 10px;
font-size: 30px;
}
</style>
</head>
<body>
<div id="game-container">
<div class="snake"></div>
<div class="food"></div>
<div class="score">0</div>
</div>
<script src="snake.js"></script>
</body>
</html>
```
CSS代码:
```css
body {
font-family: sans-serif;
}
#game-container {
width: 600px;
height: 400px;
border: 1px solid #ddd;
margin: 0 auto;
position: relative;
}
#game-container .snake, #game-container .food {
position: absolute;
width: 20px;
height: 20px;
background-color: #333;
}
#game-container .snake {
border-radius: 10px;
}
#game-container .food {
border-radius: 50%;
}
#game-container .score {
position: absolute;
top: 10px;
right: 10px;
font-size: 30px;
}
```
JavaScript代码:
```javascript
const gameContainer = document.getElementById('game-container');
const snake = document.querySelector('.snake');
const food = document.querySelector('.food');
const score = document.querySelector('.score');
const gameWidth = gameContainer.offsetWidth;
const gameHeight = gameContainer.offsetHeight;
const snakeWidth = snake.offsetWidth;
const snakeHeight = snake.offsetHeight;
const foodWidth = food.offsetWidth;
const foodHeight = food.offsetHeight;
const initialSnakeLength = 3;
const snakeSpeed = 100;
const snakeMoveDistance = 20;
let snakeX = 0;
let snakeY = 0;
let snakeDirection = 'right';
let currentScore = 0;
// 初始化贪吃蛇长度
for (let i = 0; i < initialSnakeLength; i++) {
const snakeBody = document.createElement('div');
snakeBody.classList.add('snake');
snakeBody.style.left = i * snakeMoveDistance + 'px';
gameContainer.appendChild(snakeBody);
}
// 初始化食物位置
setFoodPosition();
// 开始游戏
setInterval(moveSnake, snakeSpeed);
function moveSnake() {
// 根据方向更新贪吃蛇位置
switch (snakeDirection) {
case 'up':
snakeY -= snakeMoveDistance;
break;
case 'down':
snakeY += snakeMoveDistance;
break;
case 'left':
snakeX -= snakeMoveDistance;
break;
case 'right':
snakeX += snakeMoveDistance;
break;
}
// 判断是否撞墙
if (snakeX < 0 || snakeX + snakeWidth > gameWidth || snakeY < 0 || snakeY + snakeHeight > gameHeight) {
gameOver();
return;
}
// 判断是否吃到食物
if (isColliding(snake, food)) {
currentScore++;
score.textContent = currentScore;
setFoodPosition();
const newSnakeBody = document.createElement('div');
newSnakeBody.classList.add('snake');
gameContainer.appendChild(newSnakeBody);
}
// 移动贪吃蛇
const snakeBodies = document.querySelectorAll('.snake');
let prevX = snakeX;
let prevY = snakeY;
snakeBodies.forEach((body, index) => {
if (index === 0) {
body.style.left = snakeX + 'px';
body.style.top = snakeY + 'px';
} else {
const tempX = body.style.left;
const tempY = body.style.top;
body.style.left = prevX + 'px';
body.style.top = prevY + 'px';
prevX = tempX;
prevY = tempY;
}
});
// 判断是否撞到自己
for (let i = 1; i < snakeBodies.length; i++) {
if (isColliding(snakeBodies[0], snakeBodies[i])) {
gameOver();
return;
}
}
}
function setFoodPosition() {
const x = Math.floor(Math.random() * (gameWidth - foodWidth));
const y = Math.floor(Math.random() * (gameHeight - foodHeight));
food.style.left = x + 'px';
food.style.top = y + 'px';
}
function isColliding(a, b) {
const aRect = a.getBoundingClientRect();
const bRect = b.getBoundingClientRect();
return !(
aRect.bottom < bRect.top ||
aRect.top > bRect.bottom ||
aRect.right < bRect.left ||
aRect.left > bRect.right
);
}
function gameOver() {
alert('游戏结束,得分:' + currentScore);
window.location.reload();
}
document.addEventListener('keydown', e => {
switch (e.keyCode) {
case 37:
if (snakeDirection !== 'right') {
snakeDirection = 'left';
}
break;
case 38:
if (snakeDirection !== 'down') {
snakeDirection = 'up';
}
break;
case 39:
if (snakeDirection !== 'left') {
snakeDirection = 'right';
}
break;
case 40:
if (snakeDirection !== 'up') {
snakeDirection = 'down';
}
break;
}
});
```
这段代码实现了一个简单的贪吃蛇游戏,包括贪吃蛇的移动、食物的随机生成、得分统计和游戏结束等功能。玩家可以使用键盘上的方向键来控制贪吃蛇的移动方向。如果贪吃蛇撞墙或者撞到自己,游戏就会结束。
相关推荐
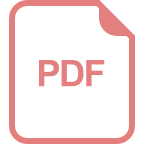











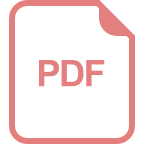
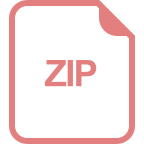
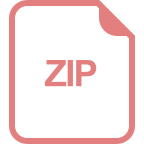