用js写一个贪吃蛇游戏
时间: 2024-05-11 15:19:07 浏览: 7
好的,以下是一个基于 JavaScript 的贪吃蛇游戏示例代码:
HTML:
```html
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇游戏</title>
<style>
canvas {
border: 1px solid #999;
}
</style>
</head>
<body>
<canvas id="canvas" width="400" height="400"></canvas>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript:
```javascript
// 获取画布和上下文
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
// 定义蛇的初始位置和大小
var snake = {
x: 160,
y: 160,
dx: 20,
dy: 0,
cells: [],
maxCells: 4,
};
// 定义食物的初始位置
var food = {
x: getRandomInt(0, 19) * 20,
y: getRandomInt(0, 19) * 20,
};
// 获取随机整数
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min)) + min;
}
// 处理键盘事件
document.addEventListener("keydown", function (e) {
// 左箭头
if (e.which === 37 && snake.dx === 0) {
snake.dx = -20;
snake.dy = 0;
}
// 上箭头
else if (e.which === 38 && snake.dy === 0) {
snake.dy = -20;
snake.dx = 0;
}
// 右箭头
else if (e.which === 39 && snake.dx === 0) {
snake.dx = 20;
snake.dy = 0;
}
// 下箭头
else if (e.which === 40 && snake.dy === 0) {
snake.dy = 20;
snake.dx = 0;
}
});
// 更新蛇的位置和长度
function updateSnake() {
snake.x += snake.dx;
snake.y += snake.dy;
// 判断是否碰到边界
if (snake.x < 0) {
snake.x = canvas.width - 20;
} else if (snake.x >= canvas.width) {
snake.x = 0;
}
if (snake.y < 0) {
snake.y = canvas.height - 20;
} else if (snake.y >= canvas.height) {
snake.y = 0;
}
// 添加新的蛇身格子
snake.cells.unshift({ x: snake.x, y: snake.y });
// 删除多余的蛇身格子
if (snake.cells.length > snake.maxCells) {
snake.cells.pop();
}
}
// 绘制蛇和食物
function draw() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 绘制蛇身格子
ctx.fillStyle = "green";
snake.cells.forEach(function (cell, index) {
ctx.fillRect(cell.x, cell.y, 20, 20);
});
// 绘制食物
ctx.fillStyle = "red";
ctx.fillRect(food.x, food.y, 20, 20);
}
// 判断是否吃到食物
function checkFood() {
if (snake.x === food.x && snake.y === food.y) {
snake.maxCells++;
food.x = getRandomInt(0, 19) * 20;
food.y = getRandomInt(0, 19) * 20;
}
}
// 游戏主循环
function main() {
setTimeout(function () {
requestAnimationFrame(main);
updateSnake();
checkFood();
draw();
}, 1000 / 15);
}
// 启动游戏
main();
```
这是一个比较简单的贪吃蛇游戏示例,你可以根据自己的需要进行修改和扩展。
相关推荐
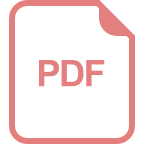













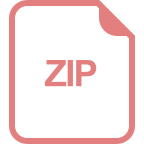