用vue写一个贪吃蛇游戏
时间: 2023-07-20 22:42:28 浏览: 72
好的,以下是一个用Vue.js写的简单贪吃蛇游戏:
HTML代码:
```html
<div id="app">
<div class="game-container">
<div class="game-board">
<div v-for="row in rows" class="game-row">
<div v-for="col in cols" :class="cellClass(row, col)"></div>
</div>
</div>
<div class="game-info">
<div class="score">
Score: {{ score }}
</div>
<button class="new-game" @click="newGame">New Game</button>
</div>
</div>
</div>
```
CSS代码:
```css
.game-container {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
}
.game-board {
display: flex;
flex-wrap: wrap;
border: 1px solid black;
}
.game-row {
display: flex;
}
.game-board div {
width: 20px;
height: 20px;
}
.snake {
background-color: green;
}
.food {
background-color: red;
}
.game-info {
margin-left: 20px;
font-size: 20px;
}
.score {
margin-bottom: 10px;
}
.new-game {
padding: 10px;
font-size: 20px;
background-color: blue;
color: white;
border: none;
cursor: pointer;
}
.new-game:hover {
background-color: darkblue;
}
```
JS代码:
```js
new Vue({
el: "#app",
data: {
rows: 20,
cols: 20,
snake: [{ row: 10, col: 10 }],
food: { row: 0, col: 0 },
direction: "right",
score: 0,
intervalId: null
},
mounted() {
this.newGame();
},
methods: {
cellClass(row, col) {
if (this.isSnake(row, col)) {
return "snake";
}
if (this.isFood(row, col)) {
return "food";
}
},
isSnake(row, col) {
return this.snake.some(cell => cell.row === row && cell.col === col);
},
isFood(row, col) {
return this.food.row === row && this.food.col === col;
},
moveSnake() {
const head = this.snake[0];
let newHead = {};
switch (this.direction) {
case "up":
newHead = { row: head.row - 1, col: head.col };
break;
case "down":
newHead = { row: head.row + 1, col: head.col };
break;
case "left":
newHead = { row: head.row, col: head.col - 1 };
break;
case "right":
newHead = { row: head.row, col: head.col + 1 };
break;
}
if (newHead.row < 0 || newHead.row >= this.rows || newHead.col < 0 || newHead.col >= this.cols) {
this.gameOver();
return;
}
if (this.isSnake(newHead.row, newHead.col)) {
this.gameOver();
return;
}
this.snake.unshift(newHead);
if (this.isFood(newHead.row, newHead.col)) {
this.score++;
this.generateFood();
} else {
this.snake.pop();
}
},
generateFood() {
let row = Math.floor(Math.random() * this.rows);
let col = Math.floor(Math.random() * this.cols);
while (this.isSnake(row, col)) {
row = Math.floor(Math.random() * this.rows);
col = Math.floor(Math.random() * this.cols);
}
this.food = { row, col };
},
gameOver() {
clearInterval(this.intervalId);
alert(`Game over! Your score is ${this.score}.`);
this.newGame();
},
newGame() {
this.snake = [{ row: 10, col: 10 }];
this.generateFood();
this.direction = "right";
this.score = 0;
clearInterval(this.intervalId);
this.intervalId = setInterval(() => {
this.moveSnake();
}, 100);
}
},
mounted() {
this.newGame();
},
created() {
window.addEventListener("keydown", event => {
switch (event.keyCode) {
case 37:
if (this.direction !== "right") {
this.direction = "left";
}
break;
case 38:
if (this.direction !== "down") {
this.direction = "up";
}
break;
case 39:
if (this.direction !== "left") {
this.direction = "right";
}
break;
case 40:
if (this.direction !== "up") {
this.direction = "down";
}
break;
}
});
}
});
```
这个贪吃蛇游戏使用了Vue.js来管理游戏状态和渲染界面。在HTML中,我们使用了v-for指令来循环渲染游戏面板上的每个格子,使用了v-bind:class指令来动态绑定每个格子的样式。在JS中,我们使用了Vue.js的data选项来存储游戏状态,使用了methods选项来定义游戏逻辑。我们通过定时器来控制蛇的移动,并且在每次移动的时候判断游戏是否结束或者是否吃到了食物。在游戏结束的时候,我们弹出一个提示框来显示得分,并且重新开始游戏。最后,我们通过Vue.js的created选项来监听键盘事件,控制蛇的方向。
相关推荐
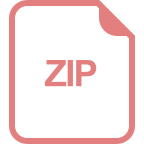
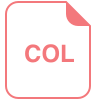
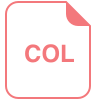
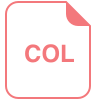
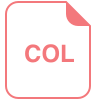
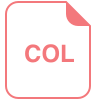









