vue.js贪吃蛇课程设计
时间: 2023-07-18 15:12:21 浏览: 64
好的,下面是一个简单的贪吃蛇游戏的Vue.js课程设计,希望能帮到你。
1. 创建项目
首先,我们需要创建一个Vue.js项目。你可以使用Vue CLI来创建项目,这里假设你已经安装了Vue CLI。
在控制台中,使用以下命令创建一个名为snake的新项目:
```
vue create snake
```
安装完成后,进入项目目录:
```
cd snake
```
启动项目:
```
npm run serve
```
现在你应该能在浏览器中看到Vue.js的欢迎页面了。
2. 创建贪吃蛇组件
接下来,我们需要创建一个贪吃蛇组件。在src/components目录下创建一个名为Snake.vue的新文件,并添加以下代码:
```
<template>
<div>
<canvas ref="canvas"></canvas>
</div>
</template>
<script>
export default {
mounted() {
const canvas = this.$refs.canvas
const context = canvas.getContext('2d')
context.fillStyle = 'black'
context.fillRect(0, 0, canvas.width, canvas.height)
}
}
</script>
<style scoped>
canvas {
border: 1px solid gray;
}
</style>
```
这个组件包含了一个canvas元素,用来绘制游戏界面。在mounted生命周期钩子中,我们获取canvas元素的引用,并使用Canvas API在画布上绘制一个黑色背景。
3. 添加游戏逻辑
现在我们需要添加一些游戏逻辑来让贪吃蛇动起来。在组件的data中添加以下变量:
```
data() {
return {
grid: 20, // 网格大小
snake: [{x: 10, y: 10}], // 蛇的初始位置
direction: 'right', // 蛇的初始方向
food: {}, // 食物的位置
score: 0 // 得分
}
}
```
这些变量用来存储游戏所需的数据,包括蛇的位置、方向、食物的位置和得分。
接下来,在组件的mounted生命周期钩子中添加以下代码:
```
mounted() {
const canvas = this.$refs.canvas
const context = canvas.getContext('2d')
context.fillStyle = 'black'
context.fillRect(0, 0, canvas.width, canvas.height)
this.generateFood()
setInterval(() => {
this.updateSnake()
this.drawSnake(context)
}, 100)
}
```
这段代码用来初始化游戏,并设置一个定时器来更新游戏状态和绘制游戏界面。
在组件的methods中添加以下方法:
```
generateFood() {
this.food = {
x: Math.floor(Math.random() * (this.$refs.canvas.width / this.grid)) * this.grid,
y: Math.floor(Math.random() * (this.$refs.canvas.height / this.grid)) * this.grid
}
},
updateSnake() {
// 计算蛇头的新位置
let newHead = {
x: this.snake[0].x,
y: this.snake[0].y
}
switch (this.direction) {
case 'up':
newHead.y -= this.grid
break
case 'down':
newHead.y += this.grid
break
case 'left':
newHead.x -= this.grid
break
case 'right':
newHead.x += this.grid
break
}
// 判断是否吃到了食物
if (newHead.x === this.food.x && newHead.y === this.food.y) {
this.snake.unshift(newHead)
this.score += 10
this.generateFood()
} else {
// 移动蛇的身体
this.snake.pop()
this.snake.unshift(newHead)
}
},
drawSnake(context) {
// 绘制蛇的身体
context.fillStyle = 'green'
this.snake.forEach((part) => {
context.fillRect(part.x, part.y, this.grid, this.grid)
})
// 绘制食物
context.fillStyle = 'red'
context.fillRect(this.food.x, this.food.y, this.grid, this.grid)
// 绘制得分
context.fillStyle = 'white'
context.font = '16px Arial'
context.fillText(`Score: ${this.score}`, 10, 20)
}
```
这些方法用来更新游戏状态、绘制游戏界面和处理用户输入。
4. 处理用户输入
最后,我们需要添加一些代码来处理用户输入。在组件的created生命周期钩子中添加以下代码:
```
created() {
window.addEventListener('keydown', (event) => {
switch (event.code) {
case 'ArrowUp':
if (this.direction !== 'down') {
this.direction = 'up'
}
break
case 'ArrowDown':
if (this.direction !== 'up') {
this.direction = 'down'
}
break
case 'ArrowLeft':
if (this.direction !== 'right') {
this.direction = 'left'
}
break
case 'ArrowRight':
if (this.direction !== 'left') {
this.direction = 'right'
}
break
}
})
}
```
这段代码用来监听用户按键事件,并根据按键来更新蛇的方向。
现在,你可以在浏览器中预览你的贪吃蛇游戏了。如果需要,你可以根据自己的需要来改进游戏,例如添加更多元素、调整游戏难度等等。
相关推荐
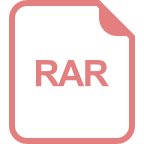
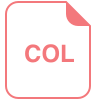
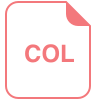
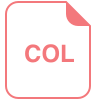
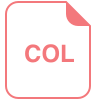
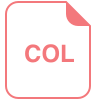









