请给我一个使用python编写一个电梯控制系统代码
时间: 2023-09-22 15:05:50 浏览: 133
### 回答1:
以下是一个使用 Python 编写的电梯控制系统代码示例:
```python
class Elevator:
def __init__(self, num_floors):
self.num_floors = num_floors
self.current_floor = 1
self.direction = "up"
self.destinations = []
def add_destination(self, floor):
if floor not in self.destinations:
self.destinations.append(floor)
if floor > self.current_floor:
self.direction = "up"
else:
self.direction = "down"
def move(self):
if self.direction == "up":
self.current_floor += 1
else:
self.current_floor -= 1
if self.current_floor in self.destinations:
self.destinations.remove(self.current_floor)
if len(self.destinations) == :
self.direction = None
def __str__(self):
return "Elevator at floor {} going {}".format(self.current_floor, self.direction)
class ElevatorControlSystem:
def __init__(self, num_elevators, num_floors):
self.elevators = [Elevator(num_floors) for i in range(num_elevators)]
def status(self):
for i, elevator in enumerate(self.elevators):
print("Elevator {} at floor {} going {}".format(i+1, elevator.current_floor, elevator.direction))
def request(self, floor):
elevator = self._find_elevator(floor)
elevator.add_destination(floor)
def step(self):
for elevator in self.elevators:
if elevator.direction:
elevator.move()
def _find_elevator(self, floor):
best_elevator = None
best_distance = 100000
for elevator in self.elevators:
if elevator.direction == "up" and floor >= elevator.current_floor and floor <= max(elevator.destinations):
distance = abs(elevator.current_floor - floor)
if distance < best_distance:
best_elevator = elevator
best_distance = distance
elif elevator.direction == "down" and floor <= elevator.current_floor and floor >= min(elevator.destinations):
distance = abs(elevator.current_floor - floor)
if distance < best_distance:
best_elevator = elevator
best_distance = distance
elif not elevator.direction:
distance = abs(elevator.current_floor - floor)
if distance < best_distance:
best_elevator = elevator
best_distance = distance
return best_elevator
```
这个电梯控制系统包括两个类:`Elevator` 和 `ElevatorControlSystem`。`Elevator` 类表示一个电梯,包括当前楼层、运行方向、目标楼层等属性和方法。`ElevatorControlSystem` 类表示整个电梯控制系统,包括多个电梯和控制方法。其中,`request` 方法用于接收外部请求,`step` 方法用于让电梯运行一步,`status` 方法用于输出当前电梯状态。
### 回答2:
下面是一个基于Python编写的简单电梯控制系统代码:
```python
class Elevator:
def __init__(self, total_floors):
self.total_floors = total_floors
self.current_floor = 1
self.direction = "up"
self.destinations = set()
def goto(self, floor):
if floor == self.current_floor:
return
self.destinations.add(floor)
if floor > self.current_floor:
self.direction = "up"
else:
self.direction = "down"
self.move()
def move(self):
while self.destinations:
if self.current_floor in self.destinations:
self.destinations.remove(self.current_floor)
print(f"Arrived at floor {self.current_floor}")
if self.direction == "up":
self.current_floor += 1
else:
self.current_floor -= 1
print(f"Moving {self.direction} to floor {self.current_floor}")
# 以下是一个简单的测试例子
elevator = Elevator(10)
elevator.goto(5)
elevator.goto(8)
elevator.goto(2)
```
这个代码创建了一个`Elevator`类,其中包含了电梯的总楼层数、当前所在楼层、运行方向和需要到达的楼层目标。`goto()`方法用于指定电梯要去的楼层,并根据当前楼层与目标楼层的关系确定运行方向。`move()`方法用于根据电梯运行方向移动,直到达到所有目标楼层。
在这个例子中,电梯总楼层数为10,然后使用`goto()`方法指定了三个不同的楼层作为目标,分别为5、8和2。最后的输出结果将显示电梯的移动过程和到达的楼层。
### 回答3:
下面是一个使用Python编写的简单电梯控制系统代码:
```python
# 定义电梯类
class Elevator:
def __init__(self):
self.current_floor = 1
self.direction = "up"
# 设置电梯当前楼层
def set_floor(self, floor):
self.current_floor = floor
# 获取电梯当前楼层
def get_floor(self):
return self.current_floor
# 设置电梯运行方向
def set_direction(self, direction):
self.direction = direction
# 获取电梯运行方向
def get_direction(self):
return self.direction
# 电梯控制系统
class ElevatorControlSystem:
def __init__(self, num_floors):
self.num_floors = num_floors
self.elevator = Elevator()
# 设置电梯当前楼层
def set_floor(self, floor):
if floor > self.num_floors or floor < 1:
print("请输入有效楼层!")
else:
self.elevator.set_floor(floor)
# 获取电梯当前楼层
def get_floor(self):
return self.elevator.get_floor()
# 设置电梯运行方向
def set_direction(self, direction):
if direction != "up" and direction != "down":
print("请输入有效方向!")
else:
self.elevator.set_direction(direction)
# 获取电梯运行方向
def get_direction(self):
return self.elevator.get_direction()
# 测试电梯控制系统
ecs = ElevatorControlSystem(10)
ecs.set_floor(5)
ecs.set_direction("up")
print("当前楼层:", ecs.get_floor())
print("当前方向:", ecs.get_direction())
```
以上代码实现了一个简单的电梯控制系统。您可以通过调用ElevatorControlSystem对象的方法来设置电梯的当前楼层和方向,并通过调用对应的方法来获取电梯的当前楼层和方向。在测试部分中,设置电梯当前楼层为5,并设置电梯方向为向上,然后输出当前楼层和方向。
相关推荐
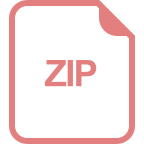
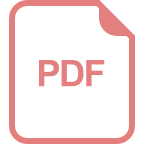














