databaseQueueWithPath 详细举例并注释
时间: 2024-03-29 14:34:15 浏览: 144
好的,下面是一个详细的示例,展示了如何使用 `databaseQueueWithPath` 方法来创建和使用 `FMDatabaseQueue` 对象,以及如何在多个线程上执行数据库查询和更新操作。
```objective-c
// 获取应用程序沙盒中的 Documents 目录
NSString *docsPath = [NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES) objectAtIndex:0];
// 拼接数据库文件的完整路径
NSString *dbPath = [docsPath stringByAppendingPathComponent:@"myDatabase.sqlite"];
// 创建 FMDatabaseQueue 对象
FMDatabaseQueue *queue = [FMDatabaseQueue databaseQueueWithPath:dbPath];
// 在主线程中执行一些数据库操作
[queue inDatabase:^(FMDatabase *db) {
// 创建一个名为 "people" 的表
[db executeUpdate:@"CREATE TABLE IF NOT EXISTS people (id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT, age INTEGER)"];
// 插入一些数据
[db executeUpdate:@"INSERT INTO people (name, age) VALUES (?, ?)", @"Tom", @(20)];
[db executeUpdate:@"INSERT INTO people (name, age) VALUES (?, ?)", @"Jane", @(25)];
}];
// 在另一个线程中执行一些数据库操作
dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{
// 在新线程中打开数据库
[queue inTransaction:^(FMDatabase *db, BOOL *rollback) {
// 查询数据
FMResultSet *result = [db executeQuery:@"SELECT * FROM people"];
// 遍历查询结果
while ([result next]) {
int id = [result intForColumn:@"id"];
NSString *name = [result stringForColumn:@"name"];
int age = [result intForColumn:@"age"];
NSLog(@"id: %d, name: %@, age: %d", id, name, age);
}
// 更新数据
[db executeUpdate:@"UPDATE people SET age = ? WHERE name = ?", @(30), @"Tom"];
}];
});
// 关闭数据库队列
[queue close];
```
在上面的示例中,我们首先获取应用程序沙盒中的 Documents 目录,并使用 `stringByAppendingPathComponent` 方法拼接出数据库文件的完整路径。然后,我们使用 `databaseQueueWithPath` 方法创建了一个 `FMDatabaseQueue` 对象,并在主线程中使用 `inDatabase` 方法执行了一些数据库操作,包括创建一个名为 "people" 的表,并插入了一些数据。
接着,我们在另一个线程中使用 `inTransaction` 方法打开了数据库,并在事务中执行了一些查询和更新操作。在查询操作中,我们使用 `executeQuery` 方法查询 "people" 表中的所有数据,并使用 `next` 方法遍历查询结果。在更新操作中,我们使用 `executeUpdate` 方法更新 "people" 表中名为 "Tom" 的记录的 age 字段值。
最后,我们使用 `close` 方法关闭了数据库队列。
希望这个示例能帮助您理解如何使用 `databaseQueueWithPath` 方法以及 `FMDatabaseQueue` 对象来执行数据库操作。
阅读全文
相关推荐
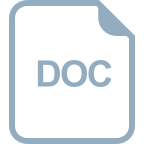
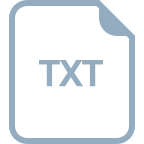
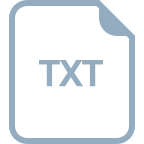
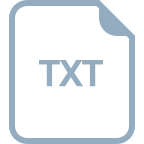
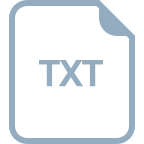
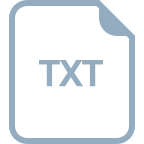
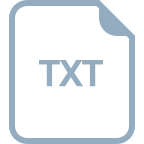
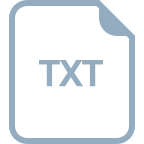
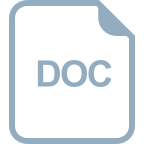
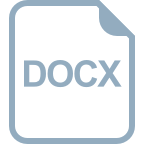
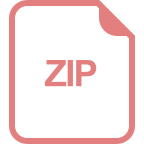
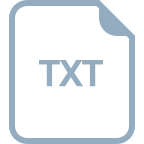
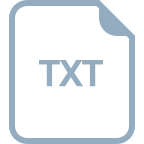
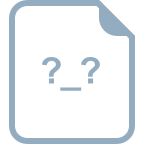