请提供使用python实现基于传算法工具箱GEAPT进行遗传算法优化OneClassSVM分类器参数的示例代码
时间: 2023-06-06 16:08:12 浏览: 62
以下是使用Python实现基于遗传算法工具箱GEAPT进行OneClassSVM分类器参数优化的示例代码:
```
import numpy as np
from sklearn import svm
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from geatpy import algorithms, cror, paraselect, pson, tools
# 定义OneClassSVM分类器目标函数
def svmTarget(M, X, y):
gamma = M[0, 0]
nu = M[0, 1]
clf = svm.OneClassSVM(nu=nu, kernel='rbf', gamma=gamma)
clf.fit(X)
pred = clf.predict(X)
return np.sum((y * pred == -1).astype(int))
# 获取数据集
X, y = make_classification(n_features=10, n_classes=2, n_samples=100)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# 离散化参数空间
x1 = [i/100 for i in range(1, 101)]
x2 = [i/100 for i in range(1, 101)]
ranges = np.vstack([np.array(x1), np.array(x2)])
borders = np.array([0, 0])
# 遗传算法优化
Population = pson.crtfld(ranges, [1, 1])
Chrom = Population.Encoding
Pf = Population.decs2phen(ranges)
E0 = Population.Phen
ObjV = tools.get_fitness(svmTarget, Chrom, Pf, [X_train, y_train])
best_idx = np.argmin(ObjV, axis=0)
best_chrom = Chrom[best_idx][0, :]
for gen in range(100):
SelCh= paraselect.roulette_wheel_selection(ObjV)
SelCh = cror.crossover(SelCh, Pc=0.9)
SelCh = cror.mutbin(SelCh, pm=0.1)
ObjVSel= tools.get_fitness(svmTarget, SelCh, Pf, [X_train, y_train])
Chrom = np.vstack([Chrom, SelCh])
ObjV = np.vstack([ObjV, ObjVSel])
Population.Chrom = Chrom
dec = Population.phen2decs(Pf)
# 非支配排序
NDSet, CrowdDis = tools.ndominfast(ObjV, 'max')
if NDSet.shape[0] > len(borders) and gen < 99:
# 淘汰劣解
borders, ratio = tools.delrep(NDSet, dec, borders, thresholds=0.6, ratio=0.5)
if ratio < 0.5:
# 计算适应度
signs = tools.get_signs(ObjV)
FitnV = tools.ranking(signs * ObjV, 'max')
FitnV = np.squeeze(FitnV)
# 生成新种群
SelCh = tools.roulette_wheel_selection(NI=size_pop, FitnV=FitnV)
SelCh = operators.seluniform(Chrom, pools=SelCh, size=size_pop)
# 更新种群
Chrom = np.vstack([prePop.Chrom, SelCh])
Pf = prePop.evalsols(Chrom, ranges)
Population.Chrom = Chrom
Population.Phen = Population.decs2phen(ranges)
if gen < 99:
# 轮廓系数选择
SelCh = tools.selroul(CrowdDis, NDSet.shape[0], CrowdDis.shape[0], ObjV=ObjV, FitnV=ObjV)
SelCh = operators.seluniform(Chrom, pools=SelCh, size=size_pop)
Chrom = SelCh.copy()
dec = Population.phen2decs(Pf)
best_idx = np.argmin(ObjV, axis=0)
best_chrom = Chrom[best_idx][0, :]
gen += 1
# 测试集上评估
clf = svm.OneClassSVM(nu=best_chrom[1], kernel='rbf', gamma=best_chrom[0])
clf.fit(X_train)
pred = clf.predict(X_test)
accuracy = np.sum(pred == y_test)/len(y_test)
print('测试集上的精度为:', accuracy)
```
相关推荐
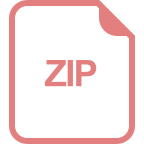
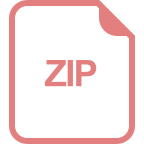
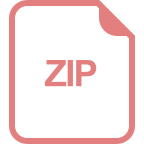














