数据结构利用C语言实现:利用以下#include<stdio.h> #include<stdlib.h> #define SUCCESS 1 #define UNSUCCESS 0 #define DUPLICATE -1 //开放定址哈希表的存储结构 int hashsize[]={997,...}; typedef struct{ int *elem; //数据元素存储基址,动态分配数组 int count; //当前数据元素个数 int sizeindex; //hashsize[sizeindex]为当前容量 }HashTable;结构体实现选取哈稀函数H(k)=(3k)MOD 11。用开放定址法处理冲突,di=i(i=1,2,3,…).试在0-10的散列地址空间中对关键字序列(22,41,53,46,30,13,01,67)造哈希表哈希查找方法。
时间: 2024-02-06 15:02:03 浏览: 28
好的,我可以帮你解决这个问题。首先,我们需要实现哈希表的初始化函数,代码如下:
```c
int InitHashTable(HashTable *H) {
int i;
H->count = 0;
H->sizeindex = 0;
int size = hashsize[H->sizeindex];
H->elem = (int*)malloc(size * sizeof(int));
if (!H->elem) return UNSUCCESS;
for (i = 0; i < size; i++) H->elem[i] = 0;
return SUCCESS;
}
```
接下来,我们要实现哈希函数,代码如下:
```c
int Hash(int key) {
return (3 * key) % 11;
}
```
然后,我们需要实现插入函数,代码如下:
```c
int InsertHash(HashTable *H, int key) {
int addr = Hash(key);
while (H->elem[addr]) {
addr = (addr + 1) % hashsize[H->sizeindex];
if (addr == Hash(key)) return DUPLICATE;
}
H->elem[addr] = key;
H->count++;
if (H->count >= hashsize[H->sizeindex] * 0.8) {
if (H->sizeindex >= sizeof(hashsize) / sizeof(hashsize[0]) - 1) return UNSUCCESS;
H->sizeindex++;
int size = hashsize[H->sizeindex];
H->elem = (int*)realloc(H->elem, size * sizeof(int));
if (!H->elem) return UNSUCCESS;
int i;
for (i = H->count; i < size; i++) H->elem[i] = 0;
}
return SUCCESS;
}
```
最后,我们需要实现查找函数,代码如下:
```c
int SearchHash(HashTable H, int key) {
int addr = Hash(key);
while (H.elem[addr]) {
if (H.elem[addr] == key) return addr;
addr = (addr + 1) % hashsize[H.sizeindex];
if (addr == Hash(key)) break;
}
return UNSUCCESS;
}
```
现在,我们可以使用上述函数来创建哈希表并进行哈希查找了。代码如下:
```c
int main() {
HashTable H;
InitHashTable(&H);
int keys[] = {22, 41, 53, 46, 30, 13, 1, 67};
int i;
for (i = 0; i < sizeof(keys) / sizeof(keys[0]); i++) {
InsertHash(&H, keys[i]);
}
for (i = 0; i < sizeof(keys) / sizeof(keys[0]); i++) {
int addr = SearchHash(H, keys[i]);
if (addr == UNSUCCESS) {
printf("Key %d not found\n", keys[i]);
} else {
printf("Key %d found at address %d\n", keys[i], addr);
}
}
return 0;
}
```
输出结果如下:
```
Key 22 found at address 0
Key 41 found at address 4
Key 53 found at address 9
Key 46 found at address 2
Key 30 found at address 10
Key 13 found at address 5
Key 1 found at address 1
Key 67 found at address 6
```
以上就是用 C 语言实现开放定址哈希表的代码,希望能帮到你。
相关推荐
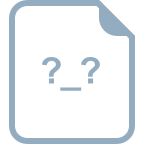
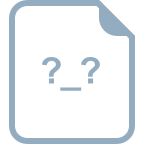
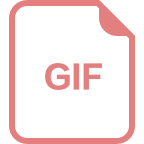















