c++编写程序模拟多个生产者和多个消费者共享缓冲池的生产和消费过程
时间: 2024-02-13 09:03:52 浏览: 42
好的,下面是一个简单的 C++ 程序,用于模拟多个生产者和多个消费者共享缓冲池的生产和消费过程:
```c++
#include <iostream>
#include <thread>
#include <vector>
#include <mutex>
#include <condition_variable>
const int BUFFER_SIZE = 5; // 缓冲池大小
std::vector<int> buffer(BUFFER_SIZE); // 缓冲池
int in = 0; // 生产者向缓冲池中添加元素的下标
int out = 0; // 消费者从缓冲池中取出元素的下标
int count = 0; // 缓冲池中元素的数量
std::mutex buffer_mutex; // 互斥锁,保护缓冲池
std::condition_variable buffer_not_full; // 缓冲池不满的条件变量
std::condition_variable buffer_not_empty; // 缓冲池不空的条件变量
void producer(int id) {
for (int i = 0; i < 10; ++i) {
std::unique_lock<std::mutex> lock(buffer_mutex);
while (count == BUFFER_SIZE) {
// 缓冲池已满,等待消费者取走元素
buffer_not_full.wait(lock);
}
buffer[in] = i;
std::cout << "Producer " << id << " produced: " << i << std::endl;
in = (in + 1) % BUFFER_SIZE;
++count;
buffer_not_empty.notify_all(); // 通知消费者缓冲池不空
}
}
void consumer(int id) {
for (int i = 0; i < 10; ++i) {
std::unique_lock<std::mutex> lock(buffer_mutex);
while (count == 0) {
// 缓冲池已空,等待生产者生产元素
buffer_not_empty.wait(lock);
}
int value = buffer[out];
std::cout << "Consumer " << id << " consumed: " << value << std::endl;
out = (out + 1) % BUFFER_SIZE;
--count;
buffer_not_full.notify_all(); // 通知生产者缓冲池不满
}
}
int main() {
std::vector<std::thread> producers;
std::vector<std::thread> consumers;
// 创建两个生产者和两个消费者
for (int i = 0; i < 2; ++i) {
producers.emplace_back(producer, i);
consumers.emplace_back(consumer, i);
}
// 等待所有生产者和消费者线程结束
for (auto& t : producers) {
t.join();
}
for (auto& t : consumers) {
t.join();
}
return 0;
}
```
该程序使用一个大小为 5 的缓冲池,多个生产者将元素添加到缓冲池中,多个消费者从缓冲池中取出元素。缓冲池满时,生产者等待缓冲池不满;缓冲池空时,消费者等待缓冲池不空。程序中使用了互斥锁和条件变量来保证线程间的同步和互斥访问。
相关推荐
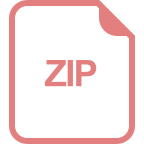
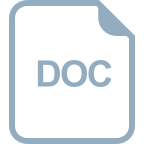














